x=tf.function(tf.float32,shape=(None,height,width,channels))
时间: 2023-07-06 18:14:36 浏览: 82
这段代码有一些问题。首先,`tf.function`函数是用来创建TensorFlow计算图的,它并不接受数据类型和形状作为参数。正确的方式应该是在函数定义时指定数据类型和形状,例如:
```python
@tf.function
def my_func(x):
# 在这里使用x,它应该是一个形状为(None, height, width, channels)的float32类型的张量
pass
```
其次,如果你想指定一个张量的形状,应该使用TensorFlow的`tf.TensorShape`类,而不是一个元组。例如:
```python
import tensorflow as tf
height = 28
width = 28
channels = 3
x = tf.Variable(tf.zeros(tf.TensorShape([None, height, width, channels]), dtype=tf.float32))
```
这将创建一个形状为(None, 28, 28, 3)的float32类型的张量,并将其赋值给变量x。你可以根据需要修改张量的形状和数据类型。
相关问题
X= tf.function(tf.float32,shape=(None,height,width,channels))怎么解决
根据你提供的代码,似乎你想要创建一个 TensorFlow 的函数,输入张量的数据类型为 `tf.float32`,形状为 `(None, height, width, channels)`。如果是这样的话,你需要使用 `tf.TensorSpec` 来指定输入张量的规格(shape 和 dtype)。
以下是一个示例代码:
```python
import tensorflow as tf
height = 10
width = 10
channels = 3
input_spec = tf.TensorSpec(shape=(None, height, width, channels), dtype=tf.float32)
@tf.function(input_signature=[input_spec])
def my_function(x):
y = tf.reduce_sum(x, axis=-1)
return y
# 使用示例
x = tf.ones((1, height, width, channels))
y = my_function(x)
print(y)
```
在上面的代码中,我们使用 `tf.TensorSpec` 定义了输入张量的形状和数据类型,并将其传递给 `tf.function`,以创建一个 TensorFlow 函数 `my_function`。`input_signature` 参数指定了输入张量的规格,以便 TensorFlow 可以在图形模式下优化该函数。
注意,我们在 `my_function` 中使用了 `tf.reduce_sum` 对输入张量的最后一维(通道数)求和,并返回结果。这只是一个示例,你可以根据你的需求修改函数的实现。
X=tf.placeholder(tf.float32,shape=(None,height,width,channels))怎么用python解决
根据你提供的代码,似乎你正在使用 TensorFlow 1.x 的 API,而 `tf.placeholder()` 是 TensorFlow 1.x 中的一个操作,用于定义输入张量的占位符。在 TensorFlow 2.x 中,我们可以直接使用张量来代替占位符。以下是一个 TensorFlow 2.x 的示例代码:
```python
import tensorflow as tf
height = 28
width = 28
channels = 1
x = tf.keras.Input(shape=(height, width, channels))
def my_function(x):
y = tf.reduce_sum(x, axis=-1)
return y
y = my_function(x)
# 使用示例
x_input = tf.ones((1, height, width, channels))
y_output = y(x_input)
print(y_output)
```
在上面的代码中,我们使用 `tf.keras.Input()` 来定义输入张量 `x`,其形状为 `(height, width, channels)`。然后,我们定义了一个函数 `my_function`,接受输入张量 `x`,并对其进行一些操作。最后,我们将输入张量 `x` 传递给函数 `my_function`,得到输出张量 `y`。
在使用模型时,我们可以将输入张量 `x_input` 传递给模型的 `__call__` 方法,得到输出张量 `y_output`。在本例中,我们使用 `tf.ones()` 来生成一个形状为 `(1, height, width, channels)` 的张量作为输入张量 `x_input`。
注意,我们在 `my_function` 中使用了 `tf.reduce_sum` 对输入张量的最后一维(通道数)求和,并返回结果。这只是一个示例,你可以根据你的需求修改函数的实现。
阅读全文
相关推荐
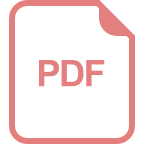
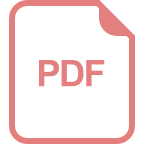
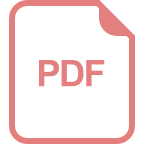

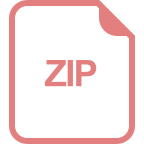
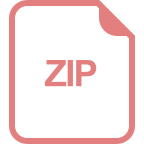
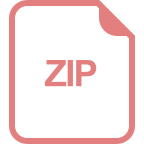
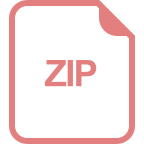
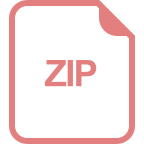
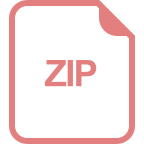
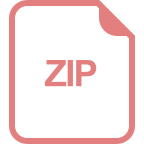
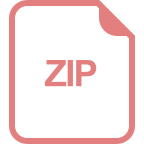
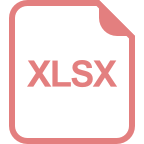
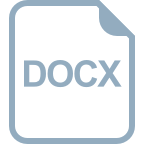
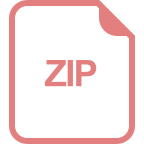