function renderImgContainer(data){ var html = ''; if(data.length == 0){ html += '<div class="layui-col-xs2">'; html += '<div class="img-box" style="text-align: center;">'; html += '<img style="width: 100px; height: 100px;" src="https://ca-1256938637.cos.ap-chengdu.myqcloud.com/school_id_1/user_id_1/20230523/%2A/none.gif">'; html += '</div>'; html += '</div>'; }else{ $.each(data,function(i,item){ html += '<div class="layui-col-xs2">'; html += '<div class="img-box" style="text-align: center;" onclick="this.classList.toggle('+"'selected"+"'"+')">'; html += '<span class="delete-img-btn">×</span>' html += '<img style="width: 100px; height: 100px;" src="'; html += item.file_url; html += '"'; html += 'id="'; html += item.file_id; html += '"'; html += 'alt="图片描述"></div>'; html += '<div style="text-align: center;">'; html += '<p class="img-title" style="cursor: pointer;overflow: hidden; text-overflow: ellipsis; white-space: nowrap;width: 100px;font-size: 5px;"'; html += 'data-file-url ='; html += item.file_url; html += '>'; html += item.file_name; html += '</p></div></div>'; }); } $('#img-container').append(html); imgPreview(); }监听delete-img-btn点击事件,并获取当前显示里面的img的id
时间: 2023-12-14 08:38:17 浏览: 32
你可以在renderImgContainer函数中添加以下代码来监听delete-img-btn的点击事件,并获取当前显示里面的img的id:
```
$(document).on('click', '.delete-img-btn', function() {
var imgId = $(this).siblings('img').attr('id');
console.log(imgId);
// 这里可以编写删除图片的代码
});
```
这里使用了jQuery的on()方法来绑定事件,因为删除按钮是动态生成的,所以需要使用事件委托方式绑定事件。当点击删除按钮时,使用siblings()方法找到对应的img元素,再使用attr()方法获取其id属性值,并将其打印出来。你可以在这里编写删除图片的代码,将imgId作为参数传入。
相关问题
<div class="layui-container"> <div class="hot-sell" v-if="bookAll.length > 0"> <div class="layui-row layui-col-space20"> <table> <tr> <td v-for="(bk,index) in bookAll" :key="index" class="layui-col-xs2" style="margin-left: 30px"> <a :href="'detail?id='+bk.bookId" class="text"> <div><img :src="'/BookShopSystem_war/' + bk.image1"></div> <p>{{bk.bookName}}</p> <p class="price">¥{{bk.bookPrice}}</p> </a> </td> </tr> </table> </div> </div> </div> <div id="houseList"></div> //列表页——分页 layui.use(['laypage'], function () { var laypage = layui.laypage; laypage.render({ elem: 'houseList' , count: 25 , limit: 5 , theme: '#daba91' , layout: ['page', 'next'] }); }); Vue.config.productionTip = false //阻止vue在启动时生成生产提示 new Vue({ el: "#app", data() { return { bookAll: [] } }, mounted() { var _this = this; axios({ method: 'get', url: 'http://localhost:8080/BookShopSystem_war/getBookAll' }).then(function (result) { var code = result.data.code; var data = result.data.data; if (code == 0) { _this.bookAll = data; } console.log(data) }) } })已省略部分代码,数据都可遍历出来,如何实现分页功能
在Vue中实现分页功能可以使用第三方库,比如element-ui和iview等,也可以使用layui等前端框架的自带分页组件。下面给出使用layui的示例代码:
HTML代码:
```
<div class="layui-container">
<div class="hot-sell" v-if="bookAll.length > 0">
<div class="layui-row layui-col-space20">
<table>
<tr>
<td v-for="(bk,index) in currentBookAll" :key="index" class="layui-col-xs2" style="margin-left: 30px">
<a :href="'detail?id='+bk.bookId" class="text">
<div><img :src="'/BookShopSystem_war/' + bk.image1"></div>
<p>{{bk.bookName}}</p>
<p class="price">¥{{bk.bookPrice}}</p>
</a>
</td>
</tr>
</table>
</div>
</div>
</div>
<div id="houseList"></div>
```
Vue代码:
```
new Vue({
el: "#app",
data() {
return {
bookAll: [],
currentBookAll: [],
page: {
count: 0,
limit: 5,
curr: 1
}
}
},
mounted() {
var _this = this;
axios({
method: 'get',
url: 'http://localhost:8080/BookShopSystem_war/getBookAll'
}).then(function (result) {
var code = result.data.code;
var data = result.data.data;
if (code == 0) {
_this.bookAll = data;
_this.page.count = Math.ceil(_this.bookAll.length / _this.page.limit);
_this.changePage(1);
}
})
},
methods: {
changePage(curr) {
var start = (curr - 1) * this.page.limit;
var end = curr * this.page.limit;
this.currentBookAll = this.bookAll.slice(start, end);
this.page.curr = curr;
layui.use(['laypage'], function () {
var laypage = layui.laypage;
laypage.render({
elem: 'houseList',
count: this.page.count,
limit: this.page.limit,
curr: this.page.curr,
theme: '#daba91',
layout: ['page', 'next'],
jump: function (obj, first) {
if (!first) {
_this.changePage(obj.curr);
}
}
});
});
}
}
})
```
上述代码中,通过计算总页数和当前页数据的起止索引,实现了分页功能。在changePage方法中,使用slice方法截取当前页的数据,并通过laypage组件渲染分页控件。在laypage的jump回调函数中,调用changePage方法更新当前页数据。
.delete-img-btn { content: "×"; display: block; position: absolute; top: 0; right: 0; width: 20px; height: 20px; color: red; text-align: center; font-size: 20px; font-weight: bold; z-index: 999; }function renderImgContainer(data){ var html = ''; if(data.length == 0){ html += '<div class="layui-col-xs2">'; html += '<div class="img-box" style="text-align: center;">'; html += '<img style="width: 100px; height: 100px;" src="https://ca-1256938637.cos.ap-chengdu.myqcloud.com/school_id_1/user_id_1/20230523/%2A/none.gif">'; html += '</div>'; html += '</div>'; }else{ $.each(data,function(i,item){ html += '<div class="layui-col-xs2">'; html += '<div class="img-box" style="text-align: center;" onclick="this.classList.toggle('+"'selected"+"'"+')">'; html += '<span class="delete-img-btn"></span>' html += '<img style="width: 100px; height: 100px;" src="'; html += item.file_url; html += '"'; html += 'id="'; html += item.file_id; html += '"'; html += 'alt="图片描述"></div>'; html += '<div style="text-align: center;">'; html += '<p class="img-title" style="cursor: pointer;overflow: hidden; text-overflow: ellipsis; white-space: nowrap;width: 100px;font-size: 5px;"'; html += 'data-file-url ='; html += item.file_url; html += '>'; html += item.file_name; html += '</p></div></div>'; }); } $('#img-container').append(html); imgPreview(); },鼠标放到图片上时,不出现红色叉号,请修正代码
您需要在CSS中添加以下样式来控制鼠标放到图片上时出现红色叉号:
```
.img-box:hover .delete-img-btn {
display: block;
}
```
这将使鼠标悬停在图像上时显示删除按钮。同时,您还需要为删除按钮添加以下CSS样式:
```
.delete-img-btn {
...
display: none; // 默认情况下不显示
}
```
这将使删除按钮在默认情况下不可见。
相关推荐
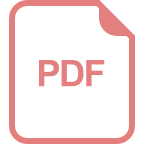
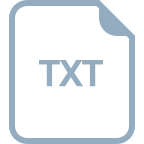
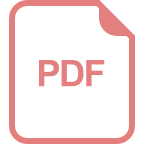

<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>图书商城-图书列表</title> <meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1"> <script src="https://cdn.bootcss.com/vue/2.5.16/vue.min.js"></script> <script src="https://unpkg.com/axios/dist/axios.min.js"></script> </head> <body> 0">
{{bk.bookName}} ¥{{bk.bookPrice}} <iframe src="footer.html" frameborder="0" scrolling="no" width="100%" height="245px"></iframe> <script src="/BookShopSystem_war/layui/layui.js"></script>这是完整的html代码,请结合你给出的分页功能整合出完整的代码











