动态规划算法(dynamic programming algorithm)
时间: 2023-09-20 16:00:38 浏览: 445
动态规划算法是一种解决问题的算法思想,它通常用于求解有重叠子问题和最优子结构的问题。通过将问题拆解为一个个相互重叠的子问题,并利用已经求解过的子问题的解来求解更大的问题,从而达到提高效率的目的。
动态规划算法的基本思想是将原问题拆解成子问题,找到子问题之间的递推关系,并利用递推关系求解子问题,最后得到原问题的解。
在使用动态规划算法求解问题时,一般需要结合状态转移方程和初始条件来定义子问题和递推关系。状态转移方程表示了子问题之间的关系,通过递推关系,可以从最简单的子问题开始,逐步求解更复杂的子问题,最终求解出整个问题的解。
动态规划算法具有自底向上的求解过程,即从最简单的子问题开始,逐步求解更复杂的子问题,直至求解出整个问题的解。这种自底向上的求解方式,避免了重复计算已经求解过的子问题,有效提高了算法的效率。
总之,动态规划算法是一种通过将问题拆解成子问题并利用子问题的解来求解更大问题的算法思想。它适用于解决有重叠子问题和最优子结构的问题,并通过递推关系和状态转移方程求解子问题,最终得到原问题的解。
相关问题
动态规划算法安排排课问题代码
Sure! Here's an example of how you can use dynamic programming to solve the course scheduling problem:
```python
def schedule_courses(courses):
n = len(courses)
dp = [0] * (n+1)
# Sort courses by end time
courses.sort(key=lambda x: x[1])
for i in range(1, n+1):
# Find the last course that doesn't conflict with the current course
j = i - 1
while j >= 0 and courses[j][1] > courses[i-1][0]:
j -= 1
# Update the maximum number of courses that can be scheduled at this point
dp[i] = max(dp[i-1], dp[j+1] + 1)
return dp[n]
```
In this code, `courses` is a list of tuples where each tuple represents a course, and the first element is the start time of the course and the second element is the end time. The goal is to schedule as many non-overlapping courses as possible.
The algorithm sorts the courses by their end times. Then, it uses dynamic programming to calculate the maximum number of courses that can be scheduled up to each course. The `dp` array stores these maximum values. The inner loop finds the last course that doesn't conflict with the current course and updates the `dp` array accordingly.
Finally, the function returns the maximum number of courses that can be scheduled.
Keep in mind that this is just a simple example, and you may need to modify it based on your specific requirements or constraints.
详细讲解一下动态规划算法、回溯算法和贪心算法
当谈到动态规划算法、回溯算法和贪心算法时,它们都是解决优化问题的经典算法。下面我会对每个算法进行详细讲解:
1. 动态规划算法(Dynamic Programming):
动态规划算法通常用于解决具有重叠子问题和最优子结构性质的问题。它通过将问题分解为子问题,并利用子问题的解来构建更大规模的问题的解。动态规划算法通常使用一个表格或数组来存储中间结果,避免重复计算。其基本思想是通过保存并重复使用子问题的解来减少计算量。
2. 回溯算法(Backtracking):
回溯算法是一种通过试错的搜索方法,用于求解满足一定条件的所有可能的解。回溯算法通过尝试每一种可能的选择并在达到不可行解时进行回溯,即返回上一层并尝试其他选择。回溯算法通常使用递归来实现,它能够穷尽所有可能的解空间,并找到满足条件的解。
3. 贪心算法(Greedy Algorithm):
贪心算法是一种通过每一步的局部最优选择来构建整体最优解的算法。贪心算法在每个步骤上都选择当前最优的解,而不考虑整体未来的结果。它通常不会回溯或重新评估之前的选择。贪心算法适用于一些特定类型的问题,如最小生成树、最短路径等,但并不适用于所有问题。
这三种算法各有优势和局限性,选择哪种算法取决于问题的性质和要求。动态规划算法通常适用于具有重叠子问题和最优子结构的问题,回溯算法适用于穷尽搜索所有可能解的问题,而贪心算法适用于局部最优解构成整体最优解的问题。在选择算法时,需要根据问题的特点和约束进行综合考虑。
阅读全文
相关推荐



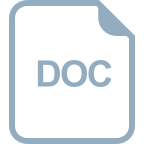
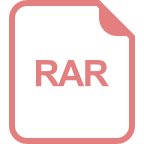

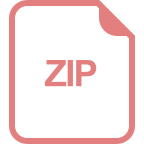

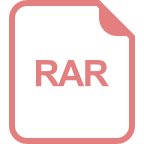







