能不能给我一个opencv的c++的yolo.h代码
时间: 2024-03-14 17:45:36 浏览: 18
以下是一个使用OpenCV C++封装的YOLOv4检测器的头文件示例:
```cpp
#ifndef YOLO_H
#define YOLO_H
#include <opencv2/opencv.hpp>
class YOLO {
public:
YOLO(const std::string& cfg_path, const std::string& weights_path, float confidence_threshold = 0.5, float nms_threshold = 0.5, int input_width = 416, int input_height = 416, const std::vector<std::string>& class_names = {});
std::vector<cv::Rect> detect(const cv::Mat& image);
std::vector<std::vector<cv::Rect>> detect_batch(const std::vector<cv::Mat>& images);
private:
cv::dnn::Net net_;
float confidence_threshold_;
float nms_threshold_;
int input_width_;
int input_height_;
std::vector<std::string> class_names_;
};
#endif // YOLO_H
```
其中包含了YOLO类的声明和定义。
使用时,可以按照以下方式进行调用:
```cpp
#include "yolo.h"
int main() {
std::string cfg_path = "/path/to/yolov4.cfg";
std::string weights_path = "/path/to/yolov4.weights";
std::vector<std::string> class_names = {"person", "car", "bicycle"};
YOLO detector(cfg_path, weights_path, 0.5, 0.5, 416, 416, class_names);
cv::Mat image = cv::imread("/path/to/image.jpg");
std::vector<cv::Rect> boxes = detector.detect(image);
for (const auto& box : boxes) {
cv::rectangle(image, box, cv::Scalar(0, 0, 255), 2);
}
cv::imshow("Detection Result", image);
cv::waitKey(0);
return 0;
}
```
在调用YOLO类的构造函数时,需要传递YOLOv4的配置文件路径和权重文件路径。还可以选择传递置信度阈值、NMS阈值、输入图像的宽度和高度以及类别名称列表。如果不传递类别名称列表,则默认使用COCO数据集的80个类别名称。
YOLO类的detect方法接受一个OpenCV Mat对象作为输入,并返回一个包含检测结果边界框的矩形向量。还可以使用detect_batch方法一次性处理多张图像并返回检测结果的向量。
相关推荐
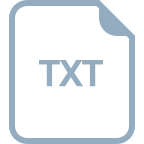
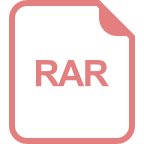














