优先级调度算法psa c++代码
时间: 2024-05-03 22:14:25 浏览: 132
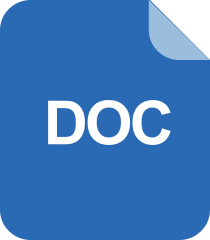
c优先调度算法代码
优先级调度算法(Priority Scheduling Algorithm)是一种常用的进程调度算法,它根据进程的优先级来确定下一个要执行的进程。优先级越高的进程,被分配的CPU时间片越多。
以下是一个使用C++实现的简单优先级调度算法的代码示例:
```cpp
#include <iostream>
#include <queue>
using namespace std;
// 进程结构体
struct Process {
int id; // 进程ID
int priority; // 进程优先级
int burstTime; // 进程执行时间
};
// 优先级比较函数,用于定义进程的优先级比较规则
struct ComparePriority {
bool operator()(const Process& p1, const Process& p2) {
return p1.priority > p2.priority;
}
};
// 优先级调度函数
void priorityScheduling(vector<Process>& processes) {
// 创建一个优先队列,按照进程的优先级进行排序
priority_queue<Process, vector<Process>, ComparePriority> pq;
// 将所有进程加入优先队列
for (const auto& process : processes) {
pq.push(process);
}
// 执行进程调度
while (!pq.empty()) {
Process currentProcess = pq.top();
pq.pop();
cout << "执行进程 " << currentProcess.id << endl;
// 执行进程的任务
for (int i = 0; i < currentProcess.burstTime; i++) {
// 模拟进程执行
}
}
}
int main() {
// 创建进程列表
vector<Process> processes = {
{1, 3, 5},
{2, 1, 3},
{3, 2, 4},
{4, 4, 2}
};
// 执行优先级调度算法
priorityScheduling(processes);
return 0;
}
```
上述代码中,我们定义了一个进程结构体 `Process`,包含进程的ID、优先级和执行时间。然后,我们使用优先队列 `priority_queue` 来按照进程的优先级进行排序。在 `priorityScheduling` 函数中,我们将所有进程加入优先队列,并按照优先级从高到低执行进程。
阅读全文
相关推荐

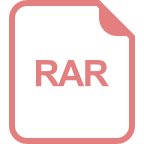
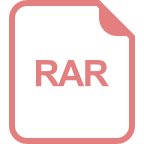
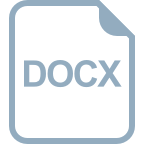
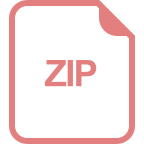
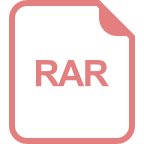
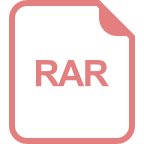
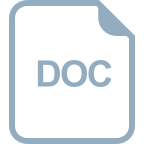
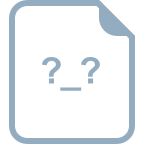
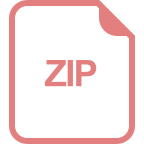
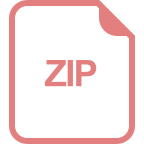