设计圆形,长方形,三角形类,并计算周长和面积,声明他们的对象数组,对象指针
时间: 2024-03-27 12:13:46 浏览: 32
好的,以下是代码实现:
```c++
#include <iostream>
#include <cmath>
using namespace std;
// 基类:图形
class Shape {
public:
virtual float getArea() = 0; // 计算面积
virtual float getPerimeter() = 0; // 计算周长
};
// 派生类:圆形
class Circle : public Shape {
private:
float radius; // 半径
public:
Circle(float r) : radius(r) {}
float getArea() { return M_PI * radius * radius; }
float getPerimeter() { return 2 * M_PI * radius; }
};
// 派生类:长方形
class Rectangle : public Shape {
private:
float length; // 长
float width; // 宽
public:
Rectangle(float l, float w) : length(l), width(w) {}
float getArea() { return length * width; }
float getPerimeter() { return 2 * (length + width); }
};
// 派生类:三角形
class Triangle : public Shape {
private:
float a; // 第一条边
float b; // 第二条边
float c; // 第三条边
public:
Triangle(float a, float b, float c) : a(a), b(b), c(c) {}
float getArea() {
float p = (a + b + c) / 2;
return sqrt(p * (p - a) * (p - b) * (p - c));
}
float getPerimeter() { return a + b + c; }
};
int main() {
// 定义对象数组
Shape* shapes[] = { new Circle(2), new Rectangle(3, 4), new Triangle(3, 4, 5) };
int n = sizeof(shapes) / sizeof(shapes[0]);
// 计算面积和周长
for (int i = 0; i < n; i++) {
cout << "Shape " << i + 1 << ":" << endl;
cout << "Area: " << shapes[i]->getArea() << endl;
cout << "Perimeter: " << shapes[i]->getPerimeter() << endl << endl;
}
// 释放内存
for (int i = 0; i < n; i++) {
delete shapes[i];
}
return 0;
}
```
在这个程序中,我们定义了一个抽象的基类 `Shape`,并分别派生出了 `Circle`、`Rectangle` 和 `Triangle` 三个类。在这些派生类中,我们重写了基类中的两个纯虚函数 `getArea()` 和 `getPerimeter()`,以计算每个图形的面积和周长。在 `main()` 函数中,我们定义了一个包含指向不同图形对象的指针的数组,并使用循环计算了每个对象的面积和周长。最后,我们释放了数组中每个对象的内存。
相关推荐
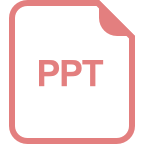














