bp神经网络代码python
时间: 2023-09-12 14:05:22 浏览: 110
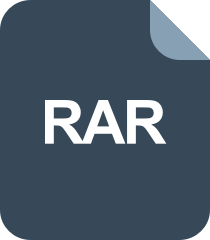
BP神经网络python代码
### 回答1:
下面是一个简单的基于Python的BP神经网络代码示例:
```python
import numpy as np
class NeuralNetwork:
def __init__(self, x, y):
self.input = x
self.weights1 = np.random.rand(self.input.shape[1],4) # 第一层权重
self.weights2 = np.random.rand(4,1) # 第二层权重
self.y = y
self.output = np.zeros(self.y.shape)
def sigmoid(self, s):
return 1/(1+np.exp(-s))
def sigmoid_derivative(self, s):
return s * (1 - s)
def feedforward(self):
self.layer1 = self.sigmoid(np.dot(self.input, self.weights1)) # 第一层输出
self.output = self.sigmoid(np.dot(self.layer1, self.weights2)) # 输出层输出
def backprop(self):
d_weights2 = np.dot(self.layer1.T, (2*(self.y - self.output) * self.sigmoid_derivative(self.output))) # 输出层误差
d_weights1 = np.dot(self.input.T, (np.dot(2*(self.y - self.output) * self.sigmoid_derivative(self.output), self.weights2.T) * self.sigmoid_derivative(self.layer1))) # 第一层误差
self.weights1 += d_weights1 # 更新第一层权重
self.weights2 += d_weights2 # 更新输出层权重
def train(self, X, y):
self.output = np.zeros(y.shape)
for i in range(1500):
self.feedforward()
self.backprop()
def predict(self, X):
self.input = X
self.feedforward()
return self.output
```
在上面的代码中,我们定义了一个名为NeuralNetwork的类,该类包含了BP神经网络的各个组成部分。其中,输入层的节点数为输入数据的特征数,输出层的节点数为1,隐藏层的节点数为4。我们使用sigmoid函数作为激活函数,并使用其导数来计算误差。在训练过程中,我们使用反向传播算法来更新权重,并重复该过程直到达到指定的迭代次数。最后,我们可以使用predict方法来对新的输入进行预测。
### 回答2:
bp神经网络是一种常用的人工神经网络模型,用于解决分类或回归问题。下面是一个简单的BP神经网络的Python代码示例:
```python
import numpy as np
class BPNeuralNetwork:
def __init__(self, input_dim, hidden_dim, output_dim, learning_rate):
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.output_dim = output_dim
self.learning_rate = learning_rate
self.weights1 = np.random.random((self.input_dim, self.hidden_dim))
self.weights2 = np.random.random((self.hidden_dim, self.output_dim))
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
def sigmoid_derivative(self, x):
return x * (1 - x)
def train(self, X, y, epochs):
for epoch in range(epochs):
hidden_layer = self.sigmoid(np.dot(X, self.weights1))
output_layer = self.sigmoid(np.dot(hidden_layer, self.weights2))
output_error = y - output_layer
output_delta = output_error * self.sigmoid_derivative(output_layer)
hidden_error = np.dot(output_delta, self.weights2.T)
hidden_delta = hidden_error * self.sigmoid_derivative(hidden_layer)
self.weights2 += np.dot(hidden_layer.T, output_delta) * self.learning_rate
self.weights1 += np.dot(X.T, hidden_delta) * self.learning_rate
def predict(self, X):
hidden_layer = self.sigmoid(np.dot(X, self.weights1))
output_layer = self.sigmoid(np.dot(hidden_layer, self.weights2))
return output_layer
```
以上代码定义了一个名为`BPNeuralNetwork`的类,构造函数中用户需要传入输入维度`input_dim`、隐藏层维度`hidden_dim`、输出维度`output_dim`和学习率`learning_rate`。`train`方法用于训练神经网络,需要传入训练数据集`X`和相应的标签`y`,以及训练的轮数`epochs`。`predict`方法用于预测输入数据`X`对应的输出。
该代码实现了前向传播和反向传播过程,通过随机初始化权重矩阵,利用梯度下降算法迭代更新权重值,以最小化预测值与实际值之间的误差。
以上是一个简单的BP神经网络的Python代码示例,实际应用中可能需要根据具体问题进行适当的调整、改进和扩展。
### 回答3:
BP神经网络是一种常用的机器学习算法,用于解决分类和回归问题。下面是一个使用Python实现的BP神经网络的简单示例代码:
```python
import numpy as np
# 定义sigmoid激活函数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 定义BP神经网络类
class BPNeuralNetwork:
def __init__(self, input_dim, hidden_dim, output_dim):
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.output_dim = output_dim
# 初始化权重和偏置
self.weights1 = np.random.randn(self.input_dim, self.hidden_dim)
self.bias1 = np.random.randn(self.hidden_dim)
self.weights2 = np.random.randn(self.hidden_dim, self.output_dim)
self.bias2 = np.random.randn(self.output_dim)
# 前向传播
def forward(self, X):
self.z1 = np.dot(X, self.weights1) + self.bias1
self.a1 = sigmoid(self.z1)
self.z2 = np.dot(self.a1, self.weights2) + self.bias2
self.a2 = sigmoid(self.z2)
return self.a2
# 反向传播
def backward(self, X, y, output):
self.error = output - y
self.delta2 = self.error * sigmoid(self.z2) * (1 - sigmoid(self.z2))
self.delta1 = np.dot(self.delta2, self.weights2.T) * sigmoid(self.z1) * (1 - sigmoid(self.z1))
self.weights2 -= self.learning_rate * np.dot(self.a1.T, self.delta2)
self.bias2 -= self.learning_rate * np.sum(self.delta2, axis=0)
self.weights1 -= self.learning_rate * np.dot(X.T, self.delta1)
self.bias1 -= self.learning_rate * np.sum(self.delta1, axis=0)
# 拟合训练数据
def fit(self, X, y, learning_rate=0.1, epochs=1000):
self.learning_rate = learning_rate
for i in range(epochs):
output = self.forward(X)
self.backward(X, y, output)
# 预测
def predict(self, X):
return np.round(self.forward(X))
```
这个简单的BP神经网络代码实现了神经网络的前向传播和反向传播过程,以及训练和预测功能。可以根据需要修改输入层、隐藏层和输出层的维度,调整学习率和训练迭代次数。
阅读全文
相关推荐

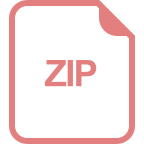
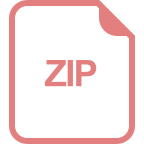







