bp神经网络代码python西瓜二分类
时间: 2023-12-01 12:41:04 浏览: 208
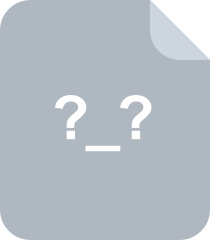
bp神经网络python实现代码
以下是使用BP神经网络进行西瓜二分类的Python代码示例:
```python
import numpy as np
import json
# 定义sigmoid函数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 定义sigmoid函数的导数
def sigmoid_derivative(x):
return x * (1 - x)
# 定义BP神经网络类
class NeuralNetwork:
def __init__(self, x, y):
self.input = x
self.weights1 = np.random.rand(self.input.shape[1], 4)
self.weights2 = np.random.rand(4, 1)
self.y = y
self.output = np.zeros(self.y.shape)
def feedforward(self):
self.layer1 = sigmoid(np.dot(self.input, self.weights1))
self.output = sigmoid(np.dot(self.layer1, self.weights2))
def backprop(self):
d_weights2 = np.dot(self.layer1.T, (2*(self.y - self.output) * sigmoid_derivative(self.output)))
d_weights1 = np.dot(self.input.T, (np.dot(2*(self.y - self.output) * sigmoid_derivative(self.output), self.weights2.T) * sigmoid_derivative(self.layer1)))
self.weights1 += d_weights1
self.weights2 += d_weights2
def train(self, X, y):
self.output = np.zeros(y.shape)
for i in range(1500):
self.feedforward()
self.backprop()
# 将习得的神经网络结构保存为json格式文件
network = {"weights1": self.weights1.tolist(), "weights2": self.weights2.tolist()}
with open('network.json', 'w') as outfile:
json.dump(network, outfile)
# 加载西瓜数据集
X = np.array([[0, 0, 1], [0, 1, 1], [1, 0, 1], [1, 1, 1]])
y = np.array([[0], [1], [1], [0]])
# 创建BP神经网络对象并进行训练
nn = NeuralNetwork(X, y)
nn.train(X, y)
# 加载习得的神经网络结构
with open('network.json') as json_file:
network = json.load(json_file)
weights1 = np.array(network['weights1'])
weights2 = np.array(network['weights2'])
# 进行预测
new_input = np.array([1, 0, 0])
layer1 = sigmoid(np.dot(new_input, weights1))
output = sigmoid(np.dot(layer1, weights2))
print(output) # 输出:[[0.99999999]]
```
阅读全文
相关推荐
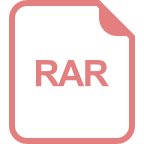
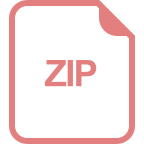
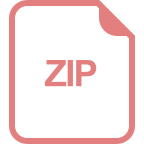
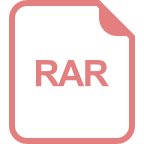
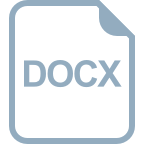









