二维自注意力机制代码keras
时间: 2023-09-13 18:08:27 浏览: 101
以下是使用Keras实现二维自注意力机制的示例代码:
```python
from keras.layers import Input, Dense, Conv2D, Flatten, Reshape, Permute, Lambda, Multiply, Add
from keras.models import Model
import keras.backend as K
def conv_block(x, filters, kernel_size, strides, padding):
x = Conv2D(filters=filters, kernel_size=kernel_size, strides=strides, padding=padding)(x)
x = Dense(units=filters, activation='relu')(x)
return x
def self_attention_block(x, filters):
# Compute the feature maps
f = conv_block(x, filters=filters, kernel_size=1, strides=1, padding='same')
g = conv_block(x, filters=filters, kernel_size=1, strides=1, padding='same')
h = conv_block(x, filters=filters, kernel_size=1, strides=1, padding='same')
# Reshape the feature maps into 2D matrices
f_flat = Flatten()(f)
g_flat = Flatten()(g)
h_flat = Flatten()(h)
# Compute the attention matrix
s = Lambda(lambda x: K.batch_dot(x[0], x[1], axes=[1, 2]))([g_flat, h_flat])
beta = Lambda(lambda x: K.softmax(x))(s)
# Apply the attention to the feature maps
o = Lambda(lambda x: K.batch_dot(x[0], x[1], axes=[1, 1]))([beta, f_flat])
o = Reshape(target_shape=(K.int_shape(x)[1], K.int_shape(x)[2], filters))(o)
# Add the original feature maps to the attended feature maps
y = Add()([o, x])
return y
# Define the input shape
input_shape = (224, 224, 3)
# Define the input layer
inputs = Input(shape=input_shape)
# Apply two self-attention blocks
x = self_attention_block(inputs, filters=64)
x = self_attention_block(x, filters=64)
# Define the output layer
outputs = Dense(units=10, activation='softmax')(x)
# Create the model
model = Model(inputs=inputs, outputs=outputs)
```
这段代码定义了一个包含两个自注意力块的卷积神经网络,输入图像的大小为224x224x3,输出10个类别的概率分布。在自注意力块中,通过三个卷积层(每个卷积层包括一个卷积操作和一个全连接层)计算出特征图f、g和h,将它们变形为2D矩阵,通过点积计算出注意力系数,然后将注意力系数应用到特征图f上,得到自注意力特征图o,与原始特征图相加得到最终的输出y。
阅读全文
相关推荐
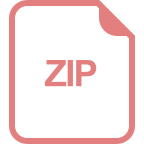
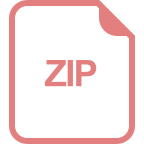


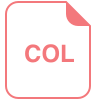
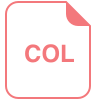
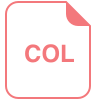
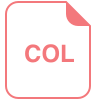
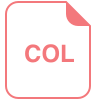
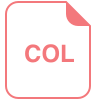
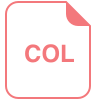
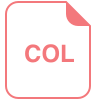
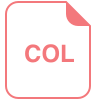



