map的key为null,value会被销毁吗
时间: 2024-04-11 19:30:55 浏览: 16
对于大多数编程语言中的 Map 数据结构来说,key 为 null 的情况是允许的。当你将一个 key 设置为 null 时,它会被存储在 Map 中,并且你可以使用该 key 获取对应的 value。然而,需要注意的是,不同的编程语言和实现可能会有不同的行为。
在一些编程语言中,当你尝试通过 null key 获取 value 时,可能会返回 null 或者抛出异常。这取决于具体的实现。如果返回 null,表示该 key 对应的 value 不存在;如果抛出异常,表示不允许使用 null 作为 key。
关于 value 的销毁问题,通常情况下,Map 数据结构并不会主动销毁 value。当你从 Map 中移除某个 key-value 对时,可能会释放对 value 的引用,但具体的内存管理还是由编程语言或者运行时环境来负责。如果没有其他引用指向该 value,那么在适当的时候,可能会由垃圾回收机制回收内存空间。
请注意,以上只是一般情况下的行为描述,并不适用于所有编程语言和实现。具体的行为还需要参考相关的文档或者查阅特定编程语言的规范。
相关问题
VxWorks软件使用map数据结构
VxWorks软件是一个实时操作系统,它提供了丰富的数据结构和功能来支持嵌入式系统开发。其中,map数据结构是一种常用的数据结构之一,用于存储键值对。
在VxWorks中,map数据结构通过sysLib库提供。使用map数据结构可以方便地存储和访问键值对数据。以下是使用map数据结构的基本步骤:
1. 创建一个map对象:
```c
MAP_ID map = mapCreate(Options);
```
这将创建一个空的map对象,并返回一个唯一的ID。
2. 向map中插入键值对:
```c
mapInsert(map, key, value);
```
这将在map中插入一个键值对,其中key是键,value是值。
3. 从map中获取值:
```c
void* pValue = mapFind(map, key);
```
这将返回与指定键关联的值,如果键不存在,则返回NULL。
4. 从map中删除键值对:
```c
mapRemove(map, key);
```
这将从map中删除指定的键值对。
5. 销毁map对象:
```c
mapDelete(map);
```
这将销毁map对象并释放相关资源。
以上是使用map数据结构的基本操作。你可以根据具体的需求来使用其他相关函数,如mapGetNext()用于遍历map中的键值对。
unordered_map c实现
unordered_map 是 C++ 标准库中的一个容器,用于存储键值对,并支持快速的插入、删除和查找操作。C++ 的 unordered_map 可以通过哈希表来实现。
要在 C 中实现一个类似 unordered_map 的数据结构,你可以使用哈希表和链表的组合。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define TABLE_SIZE 100
// 哈希表中的节点
typedef struct Node {
char key[20];
int value;
struct Node* next;
} Node;
// 哈希表
typedef struct HashMap {
Node* table[TABLE_SIZE];
} HashMap;
// 计算哈希值
int hash(const char* key) {
int hash = 0;
while (*key) {
hash += *key;
key++;
}
return hash % TABLE_SIZE;
}
// 初始化哈希表
HashMap* HashMap_Create() {
HashMap* map = (HashMap*)malloc(sizeof(HashMap));
memset(map->table, 0, sizeof(map->table));
return map;
}
// 向哈希表中插入键值对
void HashMap_Insert(HashMap* map, const char* key, int value) {
int index = hash(key);
Node* newNode = (Node*)malloc(sizeof(Node));
strcpy(newNode->key, key);
newNode->value = value;
newNode->next = NULL;
if (map->table[index] == NULL) {
map->table[index] = newNode;
} else {
Node* current = map->table[index];
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 从哈希表中删除指定键的节点
void HashMap_Remove(HashMap* map, const char* key) {
int index = hash(key);
Node* current = map->table[index];
Node* previous = NULL;
while (current != NULL && strcmp(current->key, key) != 0) {
previous = current;
current = current->next;
}
if (current == NULL) {
return; // 未找到指定键的节点
}
if (previous == NULL) {
map->table[index] = current->next;
} else {
previous->next = current->next;
}
free(current);
}
// 从哈希表中获取指定键对应的值
int HashMap_Get(HashMap* map, const char* key) {
int index = hash(key);
Node* current = map->table[index];
while (current != NULL && strcmp(current->key, key) != 0) {
current = current->next;
}
if (current == NULL) {
return -1; // 未找到指定键的节点
}
return current->value;
}
// 销毁哈希表
void HashMap_Destroy(HashMap* map) {
for (int i = 0; i < TABLE_SIZE; i++) {
Node* current = map->table[i];
while (current != NULL) {
Node* next = current->next;
free(current);
current = next;
}
}
free(map);
}
int main() {
HashMap* map = HashMap_Create();
HashMap_Insert(map, "key1", 1);
HashMap_Insert(map, "key2", 2);
HashMap_Insert(map, "key3", 3);
printf("value for key1: %d\n", HashMap_Get(map, "key1"));
printf("value for key2: %d\n", HashMap_Get(map, "key2"));
printf("value for key3: %d\n", HashMap_Get(map, "key3"));
HashMap_Remove(map, "key2");
printf("value for key2: %d\n", HashMap_Get(map, "key2"));
HashMap_Destroy(map);
return 0;
}
```
这个示例演示了如何使用哈希表和链表组合来实现一个类似 unordered_map 的数据结构。你可以根据实际需求进行修改和扩展。
相关推荐
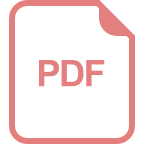
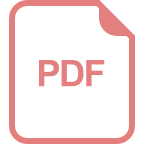









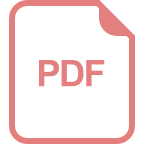
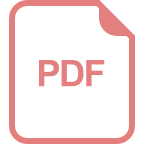
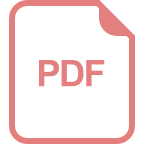