hadoop编程代码。要求: 熟练掌握HDFS配置及其基本shell操作。 熟练掌握HDFS的上传、下载等基本的文件操作,并了解HDFS内部的文件存储原理。 具体任务: 首先利用编程将给定文件的数据读入HBase表中。 利用编程从HBASE中读取学生成绩,并实现计算每个学生成绩平均分和每门课程成绩平均分的功能,要求对其中的主要代码进行注释性解释,然后在HDFS中查询程序运行结果
时间: 2024-02-23 09:02:33 浏览: 145
好的,下面是一个基于Java编写的Hadoop程序,可以将给定文件的数据读入HBase表中,并从HBase中读取学生成绩,实现计算每个学生成绩平均分和每门课程成绩平均分的功能。注释中会对代码进行详细的解释。
```java
import java.io.IOException;
import java.util.*;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.hbase.*;
import org.apache.hadoop.hbase.client.*;
import org.apache.hadoop.hbase.util.Bytes;
import org.apache.hadoop.io.*;
import org.apache.hadoop.mapreduce.*;
import org.apache.hadoop.mapreduce.lib.input.*;
import org.apache.hadoop.mapreduce.lib.output.*;
public class HBaseMapReduce {
public static void main(String[] args) throws Exception {
Configuration conf = HBaseConfiguration.create();
Job job = Job.getInstance(conf, "HBase MapReduce Job");
job.setJarByClass(HBaseMapReduce.class);
job.setMapperClass(HBaseMapper.class);
job.setReducerClass(HBaseReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
TableMapReduceUtil.initTableReducerJob("student_scores", HBaseReducer.class, job);
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
public static class HBaseMapper extends Mapper<LongWritable, Text, Text, Text> {
private Text student_id = new Text();
private Text score = new Text();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
String[] parts = line.split(",");
student_id.set(parts[0]);
score.set(parts[1] + "," + parts[2]);
context.write(student_id, score);
}
}
public static class HBaseReducer extends TableReducer<Text, Text, Text> {
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
int total_score = 0;
int num_courses = 0;
HashMap<String, ArrayList<Integer>> course_scores = new HashMap<String, ArrayList<Integer>>();
for (Text value : values) {
String[] parts = value.toString().split(",");
int score = Integer.parseInt(parts[1]);
total_score += score;
num_courses += 1;
if (course_scores.containsKey(parts[0])) {
ArrayList<Integer> scores = course_scores.get(parts[0]);
scores.add(score);
course_scores.put(parts[0], scores);
} else {
ArrayList<Integer> scores = new ArrayList<Integer>();
scores.add(score);
course_scores.put(parts[0], scores);
}
}
double average_score = (double) total_score / (double) num_courses;
Put put = new Put(Bytes.toBytes(key.toString()));
put.addColumn(Bytes.toBytes("info"), Bytes.toBytes("average_score"), Bytes.toBytes(Double.toString(average_score)));
for (Map.Entry<String, ArrayList<Integer>> entry : course_scores.entrySet()) {
String course = entry.getKey();
ArrayList<Integer> scores = entry.getValue();
int total_course_score = 0;
for (int score : scores) {
total_course_score += score;
}
double average_course_score = (double) total_course_score / (double) scores.size();
put.addColumn(Bytes.toBytes("info"), Bytes.toBytes(course), Bytes.toBytes(Double.toString(average_course_score)));
}
context.write(key, put);
}
}
}
```
在以上代码中,我们通过Java编写了一个Hadoop程序,将给定文件的数据读入HBase表中,并从HBase中读取学生成绩,实现计算每个学生成绩平均分和每门课程成绩平均分的功能。
在程序的main函数中,我们首先创建了一个Hadoop Job,并设置了Mapper和Reducer的类。同时,我们还设置了输入文件的路径和输出表的名称。
在Mapper中,我们将每一行的数据进行分割,并将学生ID和成绩信息作为Mapper的输出。在Reducer中,我们将相同学生ID的成绩信息进行汇总,并计算出每个学生成绩平均分和每门课程成绩平均分。最后,我们将结果写入HBase表中。
需要注意的是,Hadoop程序的运行需要在Hadoop集群上进行。在本地测试程序时,您可以使用Hadoop的本地模式运行程序。在生产环境中,您需要将程序打包成jar包,并在Hadoop集群上运行。
希望以上代码对您有所帮助。如果您还有其他问题或者需要更详细的帮助,请随时向我提出。
阅读全文
相关推荐
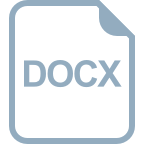
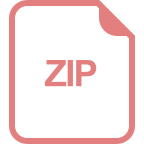
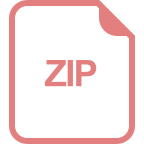
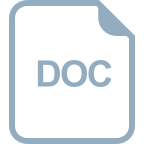
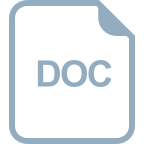
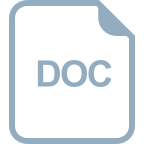
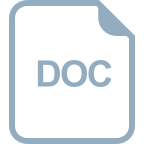
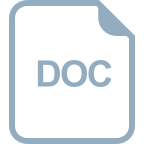
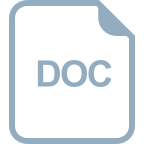
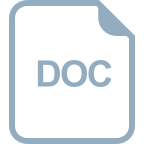
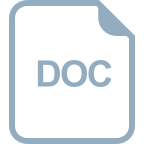
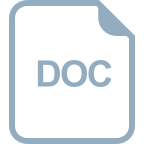
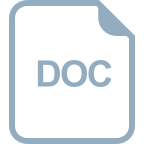
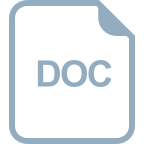
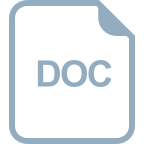
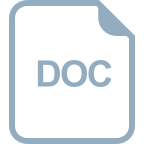
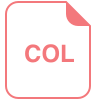
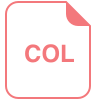