超市收银系统,多个商品的应付金额怎么加起来,请使用Python代码表达出来
时间: 2023-04-08 16:03:07 浏览: 65
可以使用以下Python代码实现多个商品的应付金额相加:
```
total = 0
while True:
price = input("请输入商品价格(输入q结束):")
if price == 'q':
break
total += float(price)
print("总金额为:", total)
```
这段代码会不断循环,让用户输入每个商品的价格,直到用户输入q结束输入。然后将每个商品的价格转换为浮点数并累加到总金额中,最后输出总金额。
相关问题
用Python写一个超市收银系统
好的,我可以为您提供一些思路来设计一个超市收银系统。首先,这个系统需要包含以下几个方面的功能:
1. 商品管理:录入商品信息(包括商品名称、商品价格、库存量等),允许修改和删除商品信息。
2. 会员管理:录入会员信息(包括会员姓名、会员等级、会员积分等),提供会员充值和积分兑换功能。
3. 收银功能:选择待结算商品,自动生成商品清单并计算总价,再根据会员等级进行折扣处理,最后生成收银小票。
4. 库存管理:对商品库存进行自动更新,及时补充和处理售罄商品。
5. 销售数据管理:记录销售数据,包括销售商品、数量、价格等信息。
以下是一个简单的Python代码实现,其中使用了面向对象编程:
```python
class Goods:
def __init__(self, name, price, stock):
self.name = name
self.price = price
self.stock = stock
class VIP:
def __init__(self, name, grade, points):
self.name = name
self.grade = grade
self.points = points
def recharge(self, amount):
self.points += amount
def exchange(self, points):
self.points -= points
class Cashier:
def __init__(self, goods_list, vip_list):
self.goods_list = goods_list
self.vip_list = vip_list
def calc_price(self, choice_list, vip):
total_price = 0
for choice in choice_list:
goods = self.goods_list[choice[0]]
total_price += goods.price * choice[1]
goods.stock -= choice[1]
if vip:
if vip.grade == 'VIP1':
total_price *= 0.9
elif vip.grade == 'VIP2':
total_price *= 0.8
elif vip.grade == 'VIP3':
total_price *= 0.7
return total_price
class Supermarket:
def __init__(self):
self.goods_list = []
self.vip_list = []
def add_goods(self, goods):
self.goods_list.append(goods)
def remove_goods(self, goods):
self.goods_list.remove(goods)
def add_vip(self, vip):
self.vip_list.append(vip)
def remove_vip(self, vip):
self.vip_list.remove(vip)
if __name__ == '__main__':
goods1 = Goods('Coca-Cola', 3, 50)
goods2 = Goods('Lay’s', 2, 80)
goods3 = Goods('Dove', 8, 20)
goods4 = Goods('Oreo', 4, 40)
goods_list = [goods1, goods2, goods3, goods4]
vip1 = VIP('张三', 'VIP1', 100)
vip2 = VIP('李四', 'VIP2', 200)
vip3 = VIP('王五', 'VIP3', 300)
vip_list = [vip1, vip2, vip3]
supermarket = Supermarket()
supermarket.add_goods(goods1)
supermarket.add_goods(goods2)
supermarket.add_goods(goods3)
supermarket.add_goods(goods4)
supermarket.add_vip(vip1)
supermarket.add_vip(vip2)
supermarket.add_vip(vip3)
cashier = Cashier(supermarket.goods_list, supermarket.vip_list)
choice_list = [(0, 2), (1, 3)]
vip = vip1
total_price = cashier.calc_price(choice_list, vip)
print('总价:', total_price)
```
这段代码中,我们定义了三个类(Goods、VIP、Cashier)表示商品、会员、收银员,并且使用一个类(Supermarket)来管理超市内的商品和会员信息。具体实现中,我们调用Cashier类的calc_price方法来计算出总价,并且传入对应的商品和会员信息。
利用python制作一个收银系统,使用字典与文本文件
好的,下面是一个简单的收银系统的代码示例,使用字典和文本文件来存储商品信息和销售记录信息:
```python
# 商品信息存储在一个字典中,键为商品编号,值为一个包含商品名称、单价和库存数量的列表
products = {
'001': ['手机', 1999, 100],
'002': ['电脑', 5999, 50],
'003': ['平板', 1499, 80],
'004': ['耳机', 99, 200],
'005': ['鼠标', 59, 300]
}
# 销售记录存储在一个文本文件中,每条记录占一行,包括商品编号、销售数量和销售时间
sales_file = 'sales.txt'
# 定义一个函数,用于更新商品库存信息和记录销售记录
def sell_product(product_id, quantity):
# 检查库存是否足够
if products[product_id][2] >= quantity:
# 更新库存信息
products[product_id][2] -= quantity
# 计算销售金额
amount = products[product_id][1] * quantity
# 记录销售记录
with open(sales_file, 'a') as f:
f.write(f'{product_id}\t{quantity}\t{amount}\t{datetime.now()}\n')
print(f'已售出 {quantity} 个 {products[product_id][0]},共计 {amount} 元。')
else:
print('库存不足,无法售出。')
# 主程序
while True:
# 显示商品列表
print('商品列表:')
print('编号\t名称\t单价\t库存')
for product_id, product_info in products.items():
print(f'{product_id}\t{product_info[0]}\t{product_info[1]}\t{product_info[2]}')
# 输入要售出的商品编号和数量
product_id = input('请输入要售出的商品编号:')
quantity = int(input('请输入要售出的数量:'))
# 售出商品
sell_product(product_id, quantity)
# 询问是否继续售出
if input('是否继续售出?(y/n)') != 'y':
break
```
注意,这只是一个简单的示例,实际应用中可能需要更复杂的逻辑和更完善的错误处理。另外,为了方便起见,本示例中使用了Tab分隔符来分隔销售记录中的字段,实际应用中可能需要使用更复杂的格式来存储销售记录。
相关推荐
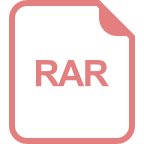
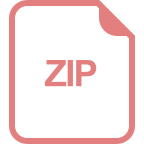
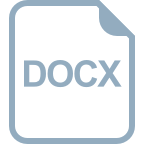












