找一个简单的车间智能排产项目进行编程复现
时间: 2023-08-01 09:11:58 浏览: 43
好的,以下是一个简单的车间智能排产项目的 Python 代码实现,基于遗传算法:
```python
import random
# 定义生产计划的类
class ProductionPlan:
def __init__(self, order_id, product_id, start_time, end_time):
self.order_id = order_id
self.product_id = product_id
self.start_time = start_time
self.end_time = end_time
self.fitness = 0
# 定义遗传算法的参数
POPULATION_SIZE = 10
CROSSOVER_RATE = 0.8
MUTATION_RATE = 0.01
ELITISM = 1
GENERATIONS = 10
# 初始化种群
def init_population(production_plans, population_size):
population = []
for i in range(population_size):
chromosome = []
for j in range(len(production_plans)):
chromosome.append(random.randint(0, 1))
population.append(chromosome)
return population
# 计算生产计划的适应度值
def calculate_fitness(production_plan):
fitness = 0
for i in range(len(production_plan)):
if production_plan[i] == 1:
fitness += 1
return fitness
# 选择适应度高的染色体
def selection(population):
population_fitness = []
for chromosome in population:
fitness = calculate_fitness(chromosome)
population_fitness.append((chromosome, fitness))
population_fitness.sort(key=lambda x: x[1], reverse=True)
selected_population = []
for i in range(ELITISM):
selected_population.append(population_fitness[i][0])
for i in range(ELITISM, len(population_fitness)):
if random.random() < CROSSOVER_RATE:
parent1 = population_fitness[i][0]
parent2 = population_fitness[random.randint(ELITISM, len(population_fitness)-1)][0]
offspring = crossover(parent1, parent2)
selected_population.append(offspring)
return selected_population
# 交叉操作
def crossover(parent1, parent2):
crossover_point = random.randint(0, len(parent1)-1)
offspring = parent1[:crossover_point] + parent2[crossover_point:]
return offspring
# 变异操作
def mutation(offspring):
for i in range(len(offspring)):
if random.random() < MUTATION_RATE:
if offspring[i] == 1:
offspring[i] = 0
else:
offspring[i] = 1
return offspring
# 遗传算法主程序
def genetic_algorithm(production_plans):
population = init_population(production_plans, POPULATION_SIZE)
for i in range(GENERATIONS):
population = selection(population)
for j in range(len(population)):
population[j] = mutation(population[j])
best_chromosome = max(population, key=lambda x: calculate_fitness(x))
return best_chromosome
# 测试
if __name__ == '__main__':
production_plans = [
ProductionPlan(1, 1, 0, 4),
ProductionPlan(2, 2, 3, 7),
ProductionPlan(3, 3, 6, 10),
ProductionPlan(4, 1, 9, 13),
ProductionPlan(5, 2, 12, 16),
ProductionPlan(6, 2, 15, 19),
ProductionPlan(7, 3, 18, 22),
ProductionPlan(8, 1, 21, 25),
ProductionPlan(9, 3, 24, 28),
ProductionPlan(10, 2, 27, 31)
]
best_chromosome = genetic_algorithm(production_plans)
print("Best chromosome:", best_chromosome)
```
这个代码实现的车间智能排产问题是:有10个订单需要生产,每个订单对应一个产品,每个产品需要一定的生产时间。我们需要找出一个生产计划,使得生产时间最短。
相关推荐
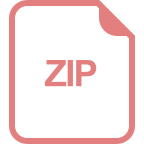
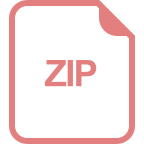














