我有一张数据表,需要根据工号字段查询出全部重复的数据,并且删除重复的数据,只保留一条,sql如何写
时间: 2023-05-11 19:06:43 浏览: 69
您好,以下是根据工号字段查询出全部重复的数据,并且删除重复的数据,只保留一条的 SQL 语句:
```
DELETE FROM table_name WHERE id NOT IN (SELECT MIN(id) FROM table_name GROUP BY job_number);
```
其中,table_name 是您的数据表名,job_number 是您的工号字段名。这条 SQL 语句会删除表中除了每个工号最小 id 对应的记录以外的所有记录,从而达到删除重复数据的目的。
相关问题
数据结构用代码建员工表,包括工号姓名月薪,输入工号可查询员工查询全部信息
好的,以下是一个示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LEN 50
#define MAX_ID_LEN 10
typedef struct Employee {
char name[MAX_NAME_LEN];
char id[MAX_ID_LEN];
float salary;
struct Employee *next;
} Employee;
Employee *head = NULL;
void addEmployee(char name[], char id[], float salary) {
Employee *newEmployee = (Employee*) malloc(sizeof(Employee));
strcpy(newEmployee->name, name);
strcpy(newEmployee->id, id);
newEmployee->salary = salary;
newEmployee->next = head;
head = newEmployee;
}
void printEmployee(Employee *employee) {
printf("Name: %s\n", employee->name);
printf("ID: %s\n", employee->id);
printf("Salary: %.2f\n", employee->salary);
}
Employee* findEmployee(char id[]) {
Employee *current = head;
while (current != NULL) {
if (strcmp(current->id, id) == 0) {
return current;
}
current = current->next;
}
return NULL;
}
void printAllEmployees() {
Employee *current = head;
while (current != NULL) {
printEmployee(current);
current = current->next;
}
}
int main() {
addEmployee("John Smith", "123456", 5000.0);
addEmployee("Mary Johnson", "789012", 4000.0);
addEmployee("David Lee", "345678", 6000.0);
char searchId[MAX_ID_LEN];
printf("Please enter an employee ID to search: ");
scanf("%s", searchId);
Employee *result = findEmployee(searchId);
if (result != NULL) {
printEmployee(result);
} else {
printf("Employee not found.\n");
}
return 0;
}
```
在这个代码中,我们使用了链表来存储员工信息,每个员工信息包括姓名、ID、月薪等属性。通过 addEmployee 函数,我们可以将新员工添加到链表的头部。通过 findEmployee 函数,我们可以根据输入的工号在链表中查找相应的员工。通过 printAllEmployees 函数,我们可以打印出所有员工的信息。在主函数中,我们通过 scanf 函数获取用户输入的工号,然后调用 findEmployee 函数查找员工信息并打印。
客服系统中 聊天记录 的数据表 需要的字段规则有哪些
一般来说,客服系统中聊天记录的数据表需要的字段规则包括:
1. ID:唯一标识每个聊天记录的编号,通常是自增长的整数。
2. 用户ID:记录与客服进行聊天的用户的唯一标识,可以是用户的用户名、手机号码等。
3. 客服ID:记录对应的客服唯一标识,可以是客服的用户名、工号等。
4. 会话ID:记录每个会话的唯一标识,用于区分不同的会话。
5. 聊天内容:记录用户和客服之间的聊天内容,通常是文本格式。
6. 发送时间:记录每条聊天记录的发送时间,通常是时间戳格式。
7. 接收时间:记录客服接收到每条聊天记录的时间,通常是时间戳格式。
8. 消息类型:记录每条聊天记录的消息类型,可以是文本、图片、语音等。
9. 消息状态:记录每条聊天记录的状态,可以是已发送、已接收、已读等。
10. 附加信息:记录一些额外的信息,如用户的IP地址、浏览器信息等。
以上是一般情况下客服系统中聊天记录的数据表需要的字段规则,具体应视实际需求而定。
相关推荐
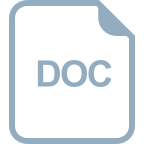
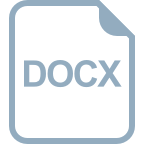
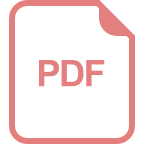












