jquery+ajax+json简单的登录注册
时间: 2023-08-08 14:07:22 浏览: 59
好的,下面是一个简单的 jQuery、Ajax 和 JSON 实现的登录注册示例:
HTML 代码:
```html
<form id="loginForm">
<input type="text" name="username" placeholder="用户名"><br>
<input type="password" name="password" placeholder="密码"><br>
<button type="submit">登录</button>
</form>
<form id="registerForm">
<input type="text" name="username" placeholder="用户名"><br>
<input type="password" name="password" placeholder="密码"><br>
<input type="password" name="confirmPassword" placeholder="确认密码"><br>
<button type="submit">注册</button>
</form>
```
jQuery 代码:
```javascript
$(function () {
// 登录表单提交
$('#loginForm').submit(function (e) {
e.preventDefault(); // 阻止表单默认提交行为
var data = $(this).serialize(); // 序列化表单数据
$.ajax({
url: '/api/login',
type: 'POST',
data: data,
dataType: 'json', // 响应数据类型为 JSON
success: function (response) {
alert(response.msg); // 弹出响应消息
if (response.code === 0) {
// 登录成功,跳转到首页
window.location.href = '/';
}
},
error: function () {
alert('网络错误,请稍后再试!');
}
});
});
// 注册表单提交
$('#registerForm').submit(function (e) {
e.preventDefault(); // 阻止表单默认提交行为
var data = $(this).serialize(); // 序列化表单数据
$.ajax({
url: '/api/register',
type: 'POST',
data: data,
dataType: 'json', // 响应数据类型为 JSON
success: function (response) {
alert(response.msg); // 弹出响应消息
if (response.code === 0) {
// 注册成功,跳转到登录页面
window.location.href = '/login';
}
},
error: function () {
alert('网络错误,请稍后再试!');
}
});
});
});
```
后端接口代码(使用 Express 框架):
```javascript
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.urlencoded({ extended: false }));
app.use(bodyParser.json());
// 模拟用户数据
let users = [
{ username: 'admin', password: 'admin123' }
];
// 登录接口
app.post('/api/login', (req, res) => {
const { username, password } = req.body;
const user = users.find(u => u.username === username && u.password === password);
if (user) {
res.json({ code: 0, msg: '登录成功!' });
} else {
res.json({ code: -1, msg: '用户名或密码错误!' });
}
});
// 注册接口
app.post('/api/register', (req, res) => {
const { username, password, confirmPassword } = req.body;
if (username && password && confirmPassword && password === confirmPassword) {
const user = users.find(u => u.username === username);
if (user) {
res.json({ code: -1, msg: '用户名已存在!' });
} else {
users.push({ username, password });
res.json({ code: 0, msg: '注册成功!' });
}
} else {
res.json({ code: -1, msg: '参数错误!' });
}
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
```
注意,这只是一个简单的示例,实际应用中还需要进行参数校验、加密等操作,以确保安全性。
相关推荐
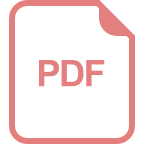
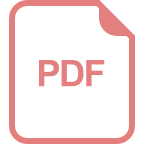














