c++ map的用法
时间: 2023-11-03 13:00:56 浏览: 240
c中的map是一种关联容器,它将键和值进行映射。在c中,使用map需要包含头文件<map>。map的用法主要包括以下几个方面:
1. 声明和初始化map:
可以使用map<键类型, 值类型> map_name;来声明一个map对象,例如:map<string, int> m;。
可以使用insert函数或者赋值运算符来初始化map,例如:m.insert(pair<string, int>("键", 值));或者m["键"] = 值;。
2. 插入元素:
可以使用insert函数来插入元素,例如:m.insert(pair<string, int>("键", 值));。
3. 查找元素:
使用find函数可以查找map中是否存在某个键,例如:map<string, int>::iterator it = m.find("键");。
如果找到了,it指向该元素;如果没有找到,it等于m.end()。
4. 遍历map:
可以使用迭代器来遍历map中的元素。例如:
map<string, int>::iterator it;
for(it = m.begin(); it != m.end(); it++){
cout<<"键="<<it->first<<" 值="<<it->second<<endl;
}
相关问题
c++map用法
C++中的`map`是一种关联容器,它将键值对(key-value pair)以有序方式存储。可以使用键来访问值,就像使用索引访问数组一样。
下面是`map`的基本使用方法:
1. 引入头文件
```c++
#include <map>
```
2. 定义`map`对象
```c++
std::map<key_type, value_type> my_map;
```
其中,`key_type`为键的类型,`value_type`为值的类型。
3. 插入元素
```c++
my_map.insert(std::make_pair(key, value));
```
或者:
```c++
my_map[key] = value;
```
4. 访问元素
```c++
value_type value = my_map[key];
```
5. 遍历`map`
```c++
for (auto it = my_map.begin(); it != my_map.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
```
其中,`it->first`表示当前键,`it->second`表示当前值。
6. 删除元素
```c++
my_map.erase(key);
```
以上就是`map`的基本使用方法,更多高级用法可以参考C++标准库文档。
c++map键值的使用方法
C++中的map是一种关联式容器,它提供了一种将键映射到值的方法。下面是map键值的使用方法:
1.定义map变量
```c++
std::map<key_type, value_type> mymap;
```
其中,key_type是键的数据类型,value_type是值的数据类型。
2.插入元素
```c++
mymap.insert(std::make_pair(key, value));
```
其中,key是键的值,value是值的值。
3.访问元素
```c++
mymap[key] = value;
```
其中,key是键的值,value是值的值。
4.遍历元素
```c++
for (auto it = mymap.begin(); it != mymap.end(); ++it) {
std::cout << it->first << " => " << it->second << '\n';
}
```
其中,it是迭代器,it->first是键的值,it->second是值的值。
阅读全文
相关推荐
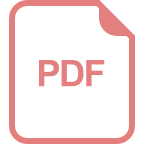
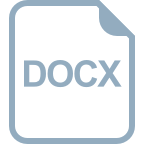
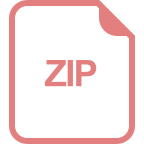
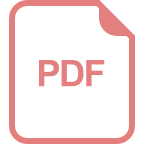
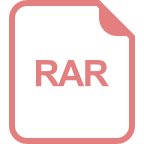
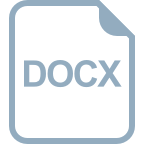
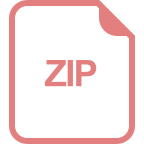
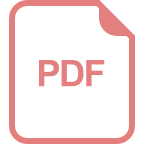
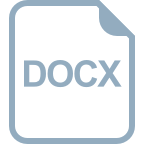
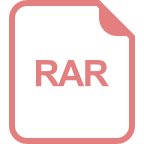
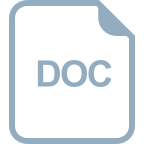




