用c语言实现线性表的插入、删除、求表长、查找给定值 e、查找第 i 个位置元素 等基本操作,要求编写的程序以菜单方式工作
时间: 2024-10-27 19:15:14 浏览: 25
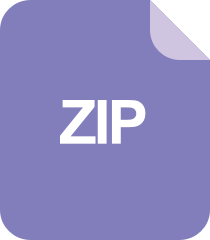
C语言实现线性表的算法与步骤
在C语言中,我们可以创建一个简单的线性表数据结构,包括动态数组作为存储容器,并提供相应的插入(Insert)、删除(Delete)、求长度(Length)、查找特定值(Search for given value)以及查找指定索引元素(Find element at index)等函数。这里是一个基本的线性表菜单驱动程序示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义线性表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化列表为空
Node* createList() {
return NULL;
}
// 在链表末尾插入元素
void insert(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
} else {
Node* temp = *head;
while (temp->next != NULL)
temp = temp->next;
temp->next = newNode;
}
}
// 删除指定值的节点
void delete(Node** head, int value) {
if (*head == NULL)
return;
if ((*head)->data == value) {
Node* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
Node* temp = *head;
while (temp->next != NULL && temp->next->data != value) {
temp = temp->next;
}
if (temp->next != NULL)
temp->next = temp->next->next;
free(temp);
}
// 求线性表的长度
int length(Node* head) {
int count = 0;
while (head != NULL) {
count++;
head = head->next;
}
return count;
}
// 查找给定值
Node* search(Node* head, int value) {
Node* current = head;
while (current != NULL) {
if (current->data == value)
return current;
current = current->next;
}
return NULL;
}
// 查找指定索引元素
int findElementAtIndex(Node* head, int index) {
Node* current = head;
int position = 0;
while (current != NULL && position < index) {
current = current->next;
position++;
}
if (position == index)
return current->data;
return -1; // 表示索引超出范围
}
int main() {
Node* listHead = createList();
int choice, value, index;
while (1) {
printf("\n1. 插入元素\n2. 删除元素\n3. 求表长\n4. 查找给定值\n5. 查找指定索引元素\n6. 退出程序\n");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("请输入值:");
scanf("%d", &value);
insert(&listHead, value);
break;
case 2:
printf("请输入要删除的值:");
scanf("%d", &value);
delete(&listHead, value);
break;
case 3:
printf("线性表的长度是:%d\n", length(listHead));
break;
case 4:
printf("请输入查找的值:");
scanf("%d", &value);
Node* foundNode = search(listHead, value);
if (foundNode)
printf("找到值 %d, 在第 %d 位\n", value, findElementIndexOf(foundNode));
else
printf("未找到该值\n");
break;
case 5:
printf("请输入索引:");
scanf("%d", &index);
int result = findElementAtIndex(listHead, index);
if (result != -1)
printf("第 %d 位的元素是:%d\n", index, result);
else
printf("索引超出范围\n");
break;
case 6:
printf("退出程序...\n");
exit(0);
default:
printf("无效选择,请重新输入。\n");
}
}
return 0;
}
```
这个程序会持续运行直到用户选择退出。你可以通过输入对应的数字来进行各种操作。
阅读全文
相关推荐
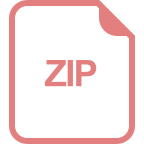
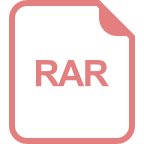

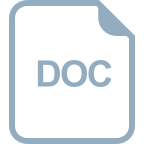
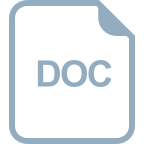
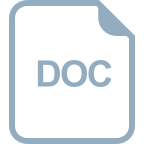
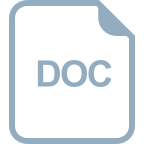
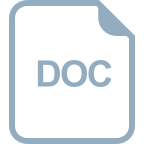
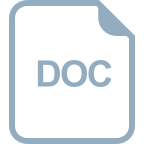
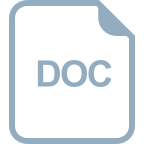
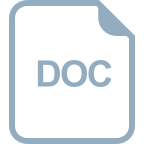

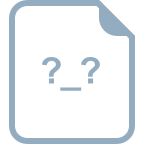
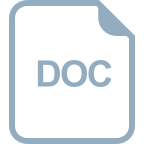
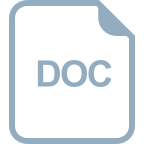
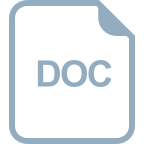
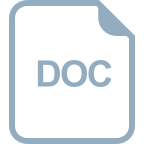
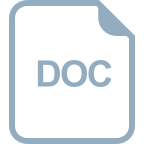
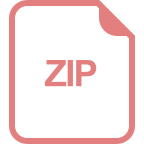