应用c语言双向链达到初始化,生成线性表3 55 2 9 7 32 66,遍历输出线性表并给出表长;将你学号后三位数值播入到第3个位置,遍历输出线性表并给出表长;将第5个元素删除,遍历输出线性表,给出表长和被删除元素。 销毁线性表,显示销毁结果的完整程序
时间: 2024-10-09 18:08:54 浏览: 0
在C语言中,我们可以使用结构体和指针来创建双向链表,并进行相应的操作。首先,我们需要定义一个双向链表节点的结构体:
```c
typedef struct Node {
int data;
struct Node* prev;
struct Node* next;
} Node;
```
接下来,我们创建一个函数来初始化链表并插入给定的数据:
```c
Node* createList(int* arr, int n) {
Node* head = NULL;
Node* tail = NULL;
for (int i = 0; i < n; i++) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = arr[i];
newNode->prev = NULL;
newNode->next = NULL;
if (head == NULL) {
head = tail = newNode;
} else {
tail->next = newNode;
newNode->prev = tail;
tail = newNode;
}
}
return head;
}
```
现在,让我们分别完成三个任务:
1. 遍历并输出线性表,以及计算长度:
```c
void printList(Node* head) {
int length = 0;
while (head != NULL) {
printf("%d ", head->data);
length++;
head = head->next;
}
printf("\nLength of the list: %d\n", length);
}
int main() {
// 初始化数据
int arr[] = {3, 55, 2, 9, 7, 32, 66};
int n = sizeof(arr) / sizeof(arr[0]);
Node* list = createList(arr, n);
// 输出原始列表
printList(list);
// 学号后三位插入
int studentNo = 123; // 示例学号
Node* thirdNode = findThirdNode(list);
thirdNode->data = studentNo % 1000; // 取后三位
// 输出更新后的列表
printList(list);
// 删除第5个元素
deleteElementAt(list, 4); // 假设从0开始计数
printList(list);
length--;
// 销毁链表
destroyList(list);
return 0;
}
```
`findThirdNode()` 和 `deleteElementAt()` 函数用于查找第3个元素的位置并删除指定位置的元素,而 `destroyList()` 函数负责释放所有节点的内存。注意,实际编写时需要添加这些辅助函数。
最后的`main()`函数会按照要求依次执行各个步骤,并打印出结果。
相关推荐
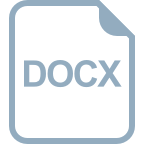
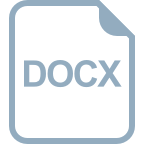
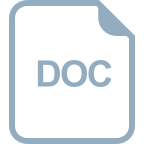









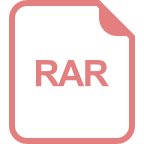
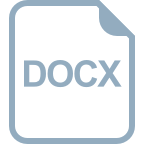
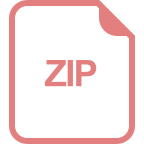
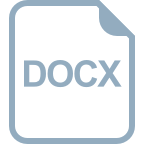
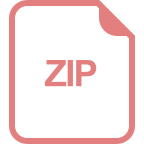
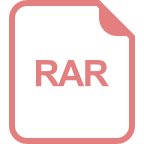
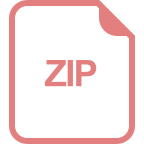