实现线性表的插入、删除、求表长、查找给定值e、查找第i个位置元素 等基本操作,要求编写的程序以菜单方式工作;
时间: 2024-11-09 20:21:45 浏览: 7
要实现线性表的基本操作,你可以使用数组或链表数据结构来存储数据。这里我将以链表为例,因为链表非常适合动态添加和删除元素。以下是一个简单的C语言程序,它实现了上述功能:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化链表头指针
Node* head = NULL;
// 插入元素到链表末尾
void insert(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
} else {
Node* temp = head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
// 删除指定值的节点(如果存在)
void delete(int value) {
Node* temp = head, *prev = NULL;
if (temp != NULL && temp->data == value) {
head = temp->next;
free(temp);
return;
}
while (temp != NULL && temp->data != value) {
prev = temp;
temp = temp->next;
}
if (temp == NULL) {
printf("Value not found in the list.\n");
return;
}
prev->next = temp->next;
free(temp);
}
// 求表长
int length() {
int count = 0;
Node* temp = head;
while (temp != NULL) {
count++;
temp = temp->next;
}
return count;
}
// 查找给定值 e
Node* find(int value) {
Node* temp = head;
while (temp != NULL) {
if (temp->data == value) {
return temp;
}
temp = temp->next;
}
return NULL;
}
// 查找第 i 个元素
Node* find_at_index(int index) {
if (index >= 0 && index < length()) {
Node* temp = head;
for (int i = 0; i < index; i++) {
temp = temp->next;
}
return temp;
} else {
printf("Index out of range.\n");
return NULL;
}
}
// 主菜单
void menu() {
while (true) {
printf("\n1. Insert\n2. Delete\n3. Check Length\n4. Find Value\n5. Find Element at Index\n6. Exit\n");
int choice;
scanf("%d", &choice);
switch (choice) {
case 1:
int value;
printf("Enter value to insert: ");
scanf("%d", &value);
insert(value);
break;
case 2:
int value_to_delete;
printf("Enter value to delete: ");
scanf("%d", &value_to_delete);
delete(value_to_delete);
break;
case 3:
printf("List length: %d\n", length());
break;
case 4:
int search_value;
printf("Search value: ");
scanf("%d", &search_value);
Node* result = find(search_value);
if (result) {
printf("Found at position: %ld\n", (long)(result - head + 1));
} else {
printf("Value not found.\n");
}
break;
case 5:
int index;
printf("Enter index: ");
scanf("%d", &index);
Node* result = find_at_index(index);
if (result) {
printf("Element at index: %d\n", result->data);
} else {
printf("Invalid index.\n");
}
break;
case 6:
printf("Exiting...\n");
exit(0);
default:
printf("Invalid choice. Try again.\n");
}
}
}
int main() {
menu();
return 0;
}
```
这个程序首先定义了链表节点结构,然后创建了一个主菜单函数,根据用户的输入执行相应的操作。每个操作都有相应的函数来处理实际的操作,比如插入、删除、查找等。
阅读全文
相关推荐
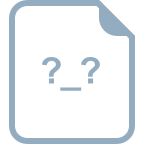
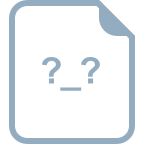
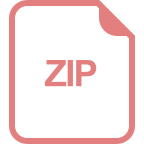














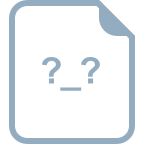
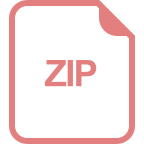