class T_Adapter(nn.Module): def __init__(self, D_features, mlp_ratio=0.25, act_layer=nn.GELU): super().__init__() D_hidden_features = int(D_features * mlp_ratio) self.D_fc1 = nn.Linear(D_features, D_hidden_features) self.D_fc2 = nn.Linear(D_hidden_features, D_features) self.act = act_layer() def forward(self, x): xs = self.D_fc1(x) xs = self.act(xs) xs = self.D_fc2(xs) x = xs # x = rearrange(x, 'B N T D -> (B T) N D') return x
时间: 2023-06-24 21:08:42 浏览: 51
这段代码是一个PyTorch模块的定义,称为 T_Adapter。它包含一个前向传递函数forward(x),其中x是输入张量。T_Adapter由两个完全连接的层组成:D_fc1和D_fc2层,其输入和输出维度为D_features和D_hidden_features,和D_features和D_features,分别。这两层之间使用激活函数act_layer()进行非线性变换。整个模块的目的是对输入进行特征降维,即从D_features到D_hidden_features,然后再将其恢复到D_features。这通常用于将预先训练好的模型应用于新的任务时,调整模型以适应新的输入特征分布。
相关问题
class Mlp(nn.Module): def __init__(self, in_features, hidden_features=None, out_features=None, act_layer=nn.GELU, drop=0.): super().__init__() out_features = out_features or in_features hidden_features = hidden_features or in_features self.fc1 = nn.Linear(in_features, hidden_features) self.act = act_layer() self.fc2 = nn.Linear(hidden_features, out_features) self.drop = nn.Dropout(drop)是什么意思
这段代码定义了一个多层感知机(Multi-Layer Perceptron,MLP)的神经网络模型。下面是每个部分的解释:
- `class Mlp(nn.Module):`:定义了一个名为`Mlp`的类,并继承自`nn.Module`,这意味着它是一个PyTorch模型。
- `def __init__(self, in_features, hidden_features=None, out_features=None, act_layer=nn.GELU, drop=0.):`:定义了类的初始化函数,用于初始化模型的参数。
- `super().__init__()`:调用父类(`nn.Module`)的初始化函数。
- `out_features = out_features or in_features`和`hidden_features = hidden_features or in_features`:如果未指定输出特征和隐藏特征的数量,则将它们设置为输入特征的数量。
- `self.fc1 = nn.Linear(in_features, hidden_features)`:定义了一个全连接层(`nn.Linear`),它将输入特征映射到隐藏特征。
- `self.act = act_layer()`:定义激活函数层,这里使用的是`act_layer`参数指定的激活函数(默认为`nn.GELU`)。
- `self.fc2 = nn.Linear(hidden_features, out_features)`:定义了另一个全连接层,它将隐藏特征映射到输出特征。
- `self.drop = nn.Dropout(drop)`:定义了一个Dropout层,用于在训练过程中随机丢弃一部分神经元,以减少过拟合风险。
这段代码的作用是创建一个MLP模型,并定义了模型的结构和参数。具体的使用方式需要根据实际情况进行调用和训练。
class SAdapter2(nn.Module): def __init__(self, D_features, mlp_ratio=0.25, act_layer=nn.GELU): super().__init__() D_hidden_features = int(D_features * mlp_ratio) self.D_fc1 = nn.Linear(D_features, D_hidden_features) self.D_fc2 = nn.Linear(D_hidden_features, D_features) self.act = act_layer() def forward(self, x): xs = self.D_fc1(x) xs = self.act(xs) xs = self.D_fc2(xs) x = x + xs return x
这段代码定义了一个叫做 SAdapter2 的神经网络模块,它是 nn.Module 的子类,因此可以方便地和其它模块组合。该模块的输入是一个大小为 D_features 的张量 x,输出也是一个大小为 D_features 的张量 x,但是经过了一定的变换。
在 __init__ 方法中,该模块定义了两个全连接层 self.D_fc1 和 self.D_fc2,它们分别将输入张量 x 经过线性变换,变成了一个大小为 D_hidden_features 的张量,然后经过激活函数 act_layer 得到一个新的张量 xs,最后再经过一次线性变换,将该张量恢复到大小为 D_features。其中,D_hidden_features 是一个超参数,表示中间的隐藏层的维度,mlp_ratio 表示隐藏层维度和输入维度的比例,act_layer 表示激活函数,默认为 GELU。
在 forward 方法中,该模块将输入张量 x 经过第一个全连接层 self.D_fc1 得到一个新的张量 xs,然后经过激活函数得到一个新的张量 xs,接着再经过第二个全连接层 self.D_fc2 得到一个新的张量 xs,最后将 x 和 xs 相加得到新的张量 x,返回该张量。
总之,这个模块可以看做是一种残差连接的形式,通过在输入和输出之间添加一些线性变换和非线性变换,来让神经网络更加深层次,提高模型的表达能力。
相关推荐
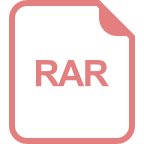
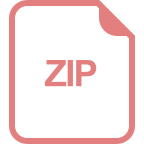













