pytorch view
时间: 2023-10-21 17:13:59 浏览: 43
PyTorch中的view函数用于改变一个Tensor的形状(shape),也就是对Tensor进行reshape操作。它返回一个新的Tensor,而不是修改原来的Tensor。
下面是view函数的基本用法:
```python
new_tensor = tensor.view(new_shape)
```
其中,`tensor`是要进行reshape操作的Tensor,`new_shape`是指定的新形状,`new_tensor`是返回的新Tensor。
需要注意的是,`new_shape`中的元素个数必须和原来的Tensor中元素个数相同,否则会抛出运行时异常。如果`new_shape`中有一个维度为-1,则表示这个维度的大小由其他维度来推导。
下面是一个简单的例子:
```python
import torch
x = torch.randn(2, 3, 4)
print(x.shape)
# 改变x的形状,变成一个大小为(2, 12)的Tensor
y = x.view(2, 12)
print(y.shape)
# 改变x的形状,自动推导第二个维度的大小
z = x.view(2, -1)
print(z.shape)
```
输出结果为:
```
torch.Size([2, 3, 4])
torch.Size([2, 12])
torch.Size([2, 12])
```
相关问题
pytorch view
在PyTorch中,view函数的作用是重构张量的维度,类似于NumPy中的resize()功能。\[1\]通过使用view函数,可以改变张量的形状,而不改变其元素的数量。view函数的参数可以是一个或多个整数,用于指定新张量的形状。如果某个参数为-1,则表示该维度取决于其他维度,PyTorch会自动计算并补充该维度的大小。\[2\]另外,如果view函数的参数为-1,则表示将张量转换为一维张量。\[3\]通过使用view函数,可以方便地改变张量的形状,以适应不同的计算需求。
#### 引用[.reference_title]
- *1* [pytorch--view函数用法](https://blog.csdn.net/weixin_41978551/article/details/111265075)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* *3* [详解Pytorch中的view函数](https://blog.csdn.net/qq_40968179/article/details/128074940)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
pytorch view as
PyTorch's `view_as` function is used to reshape a tensor to the shape of another tensor, which is useful when you want to perform operations that require tensors of the same shape. It returns a new tensor with the same data as the input tensor but with the desired shape.
Here's an example usage:
```python
import torch
# Create a tensor
x = torch.tensor([[1, 2, 3], [4, 5, 6]])
# Create a target tensor with the desired shape
target_shape = torch.tensor([2, 3])
# Reshape x to match the target shape using view_as
reshaped_x = x.view_as(target_shape)
print(reshaped_x)
```
Output:
```
tensor([[1, 2, 3],
[4, 5, 6]])
```
In this example, `view_as` reshapes the tensor `x` to match the shape of `target_shape`, which is `[2, 3]`. The resulting tensor `reshaped_x` has the same values as `x`, but its shape is now `[2, 3]`.
相关推荐
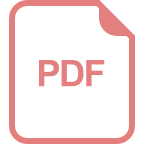
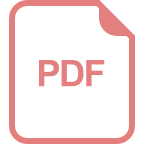













