lstm注意力机制代码
时间: 2023-09-28 15:09:45 浏览: 81
下面是一个简单的LSTM+注意力机制的代码实现:
```
import tensorflow as tf
from tensorflow.contrib.rnn import LSTMCell
class AttentionLSTM(object):
def __init__(self, num_units, max_seq_len, input_dim, output_dim):
self.num_units = num_units
self.max_seq_len = max_seq_len
self.input_dim = input_dim
self.output_dim = output_dim
self.inputs = tf.placeholder(tf.float32, shape=[None, max_seq_len, input_dim])
self.labels = tf.placeholder(tf.float32, shape=[None, output_dim])
self.seq_len = tf.placeholder(tf.int32, shape=[None])
self.W_omega = tf.Variable(tf.random_uniform([self.num_units, self.num_units], -1.0, 1.0))
self.b_omega = tf.Variable(tf.random_uniform([self.num_units], -1.0, 1.0))
self.u_omega = tf.Variable(tf.random_uniform([self.num_units], -1.0, 1.0))
self.lstm_cell = LSTMCell(num_units=self.num_units)
outputs, state = tf.nn.dynamic_rnn(self.lstm_cell, self.inputs, dtype=tf.float32, sequence_length=self.seq_len)
# Attention mechanism
v = tf.tanh(tf.matmul(tf.reshape(outputs, [-1, self.num_units]), self.W_omega) + tf.reshape(self.b_omega, [1, -1]))
vu = tf.matmul(v, tf.reshape(self.u_omega, [-1, 1]))
exps = tf.reshape(tf.exp(vu), [-1, self.max_seq_len])
alphas = exps / tf.reshape(tf.reduce_sum(exps, 1), [-1, 1])
output = tf.reduce_sum(outputs * tf.reshape(alphas, [-1, self.max_seq_len, 1]), 1)
self.logits = tf.layers.dense(output, self.output_dim, activation=None)
self.loss = tf.losses.softmax_cross_entropy(self.labels, self.logits)
self.optimizer = tf.train.AdamOptimizer().minimize(self.loss)
```
在这个代码中,我们首先定义了输入和标签的placeholder,以及序列长度的placeholder。然后定义注意力机制中的参数,W_omega、b_omega和u_omega。接下来,我们使用LSTMCell定义了一个LSTM单元,并使用dynamic_rnn函数对其进行了封装。在LSTM的输出中,我们使用了注意力机制。具体地,我们首先计算了v,然后计算了u,最后计算了alphas。最后,我们使用alphas作为权重,对LSTM的输出进行加权求和,得到了最终的输出。最后,我们使用一个全连接层将输出转换为标签,并计算了交叉熵损失和Adam优化器。
阅读全文
相关推荐
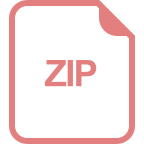

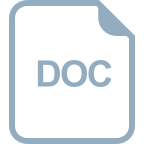


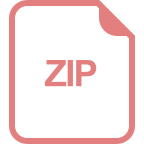
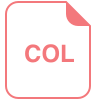








