解释这段代码 public int[] read(String filename,int a[]){ File file=new File(filename); try { Scanner input=new Scanner(file); int i=0; while(input.hasNext()){ a[i]=input.nextInt(); i++; } } catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } return a; }
时间: 2023-06-02 15:08:13 浏览: 42
这段代码是一个读取文件并将文件中的数字存入数组的方法。方法中需要传入一个文件名和一个整数数组,方法返回值为整数数组。方法将文件名转换为一个File对象,使用Scanner类读取文件内容,并将读取到的整数存入整数数组中。如果文件不存在,会抛出FileNotFoundException异常。
相关问题
补全以下代码import java.io.*; public class CopyFile extends Thread{ private String sourcFilePaht;//复制原文件的路径和文件名,如“c:\\ss.rar private String targetFilePaht;// 复制目标文件的路径和文件名,如“d:\\ss.rar public CopyFile(String sourcFilePaht, String targetFilePaht) { super(); this.sourcFilePaht = sourcFilePaht; this.targetFilePaht = targetFilePaht; } public CopyFile() { super(); } //覆写Thread类的run方法 { try { File file1=new File(sourcFilePaht); String filename=file1.getName(); File file2=new File(targetFilePaht); FileInputStream fis=new FileInputStream(file1); BufferedInputStream bis= ; FileOutputStream fos=new FileOutputStream(file2); BufferedOutputStream bos= ; byte bytes[]=new byte[2*1024]; int len=0; while((len= )!=-1){ ; bos.flush(); } bos.close(); fos.close(); bis.close(); fis.close(); System.out.println(filename+"文件复制成功!"); } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); System.out.println("文件复制失败!"); } } }
好的,下面是补全后的代码:
```
import java.io.*;
public class CopyFile extends Thread {
private String sourcFilePaht;//复制原文件的路径和文件名,如“c:\\ss.rar
private String targetFilePaht;// 复制目标文件的路径和文件名,如“d:\\ss.rar
public CopyFile(String sourcFilePaht, String targetFilePaht) {
super();
this.sourcFilePaht = sourcFilePaht;
this.targetFilePaht = targetFilePaht;
}
public CopyFile() {
super();
}
//覆写Thread类的run方法
public void run() {
try {
File file1 = new File(sourcFilePaht);
String filename = file1.getName();
File file2 = new File(targetFilePaht);
FileInputStream fis = new FileInputStream(file1);
BufferedInputStream bis = new BufferedInputStream(fis);
FileOutputStream fos = new FileOutputStream(file2);
BufferedOutputStream bos = new BufferedOutputStream(fos);
byte bytes[] = new byte[2*1024];
int len = 0;
while((len = bis.read(bytes)) != -1){
bos.write(bytes, 0, len);
bos.flush();
}
bos.close();
fos.close();
bis.close();
fis.close();
System.out.println(filename + "文件复制成功!");
} catch (Exception e) {
e.printStackTrace();
System.out.println("文件复制失败!");
}
}
}
```
在上面的代码中,`CopyFile` 类继承了 `Thread` 类,并重写了 `run()` 方法,用来实现文件复制的功能。在 `run()` 方法中,首先根据传入的原文件路径和目标文件路径创建相应的 `File` 对象,然后使用字节缓冲输入输出流实现文件复制的功能。复制完成后,输出复制成功的信息。
需要注意的是,在使用字节缓冲输入输出流时,要先创建相应的输入输出流对象,然后再将它们作为参数传入到字节缓冲输入输出流的构造方法中,如下面的代码所示:
```
FileInputStream fis = new FileInputStream(file1);
BufferedInputStream bis = new BufferedInputStream(fis);
FileOutputStream fos = new FileOutputStream(file2);
BufferedOutputStream bos = new BufferedOutputStream(fos);
```
在复制文件的过程中,需要不断读取原文件中的数据,并将其写入到目标文件中,直到读取到文件末尾,如下面的代码所示:
```
while((len = bis.read(bytes)) != -1){
bos.write(bytes, 0, len);
bos.flush();
}
```
最后,在关闭输入输出流对象时,需要先关闭字节缓冲输入输出流对象,然后再关闭相应的输入输出流对象,如下面的代码所示:
```
bos
解释这段代码package question7; import java.io.DataOutputStream; import java.io.File; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.PrintWriter; import java.util.ArrayList; import java.util.HashSet; import java.util.Scanner; import java.util.Set; import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; import java.util.Random; public class Test { public static void main(String args[]){ Test test=new Test(); Scanner input=new Scanner(System.in); String s1=new String(); s1=input.nextLine(); String[] s2 = s1.split(" "); int[] l=new int[s2.length]; for(int i=0 ; i<s2.length;i++){ l[i]=Integer.parseInt(s2[i]); } test.write("test.txt", l); int[] readlist=test.read("test.txt",l); isPrime isprime=new isPrime(readlist); for(int i=1;i<=10;i++){ new Thread(isprime).start(); } try { Thread.sleep(1000); //1000 毫秒,也就是1秒. } catch(InterruptedException ex) { Thread.currentThread().interrupt(); } Set<Integer> set=new HashSet(); set=isprime.getSet(); System.out.println("素数set"+set); System.out.println("输入查询数字"); int num=input.nextInt(); if(set.contains(num)){ System.out.println(num+"是素数"); } else System.out.println(num+"不是是素数"); } public void write(String filename,int a[]){ File file = new File(filename); try { PrintWriter output=new PrintWriter(file); for(int i=0;i<a.length;i++){ output.print(a[i]+" "); } output.close(); } catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public int[] read(String filename,int a[]){ File file=new File(filename); try { Scanner input=new Scanner(file); int i=0; while(input.hasNext()){ a[i]=input.nextInt(); i++; } } catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } return a; } }
这段代码是一个 Java 程序,主要功能是读取用户输入的一串整数,将其写入文件 test.txt,然后又从这个文件中读取整数列表,并查找出其中的素数并存储在一个 HashSet 中,最后判断用户输入的数字是否为素数。具体实现包括定义了一个 isPrime 类用于判断一个数字是否为素数,使用了多线程来处理多个数字的判断,使用了 HashSet 来存储素数列表。
相关推荐
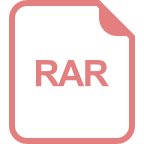
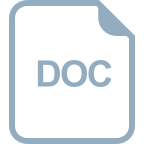
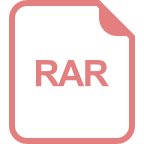













