基学习器为lnn的boosting五分类代码
时间: 2024-03-27 22:36:51 浏览: 14
好的,以下是基学习器为LNN的五分类Boosting代码示例(Python实现):
```python
import numpy as np
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Sigmoid函数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# LNN的定义
class LNN:
def __init__(self, input_dim, learning_rate=0.01):
self.weights = np.random.randn(input_dim, 1)
self.bias = np.random.randn(1)
self.lr = learning_rate
# 训练一个基学习器
def train(self, X_train, y_train, iterations):
for i in range(iterations):
# 前向传播
z = np.dot(X_train, self.weights) + self.bias
a = sigmoid(z)
# 反向传播
dz = a - y_train.reshape(-1, 1)
dw = np.dot(X_train.T, dz)
db = np.sum(dz)
# 更新权重和偏置
self.weights -= self.lr * dw
self.bias -= self.lr * db
# 预测
def predict(self, X_test):
z = np.dot(X_test, self.weights) + self.bias
a = sigmoid(z)
return np.round(a).astype(int)
# 定义一个Boosting分类器
class BoostingClassifier:
def __init__(self, base_estimator, n_estimators):
self.base_estimator = base_estimator
self.n_estimators = n_estimators
self.estimators = []
# 训练模型
def fit(self, X, y):
# 初始化权重
sample_size = X.shape[0]
weights = np.ones(sample_size) / sample_size
for i in range(self.n_estimators):
# 基于样本权重抽取样本
indices = np.random.choice(sample_size, sample_size, p=weights)
# 训练一个基学习器
estimator = self.base_estimator(input_dim=X.shape[1])
estimator.train(X[indices], y[indices], iterations=100)
# 记录基学习器,并更新样本权重
self.estimators.append(estimator)
y_pred = estimator.predict(X)
error = np.sum(weights * (y != y_pred))
alpha = np.log((1 - error) / error) / 2
weights = weights * np.exp(-alpha * y * y_pred)
# 预测
def predict(self, X):
y_pred = np.zeros(X.shape[0])
for estimator in self.estimators:
y_pred += estimator.predict(X).reshape(-1) * alpha
return np.round(y_pred).astype(int)
# 生成样本数据
X, y = make_classification(n_samples=1000, n_features=20, n_informative=10, n_classes=5, random_state=42)
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 定义基学习器
base_estimator = LNN
# 定义Boosting分类器
clf = BoostingClassifier(base_estimator=base_estimator, n_estimators=100)
# 训练模型
clf.fit(X_train, y_train)
# 预测测试集结果
y_pred = clf.predict(X_test)
# 输出模型准确率
print("Accuracy:", accuracy_score(y_test, y_pred))
```
在这个例子中,我们使用LNN作为基学习器,并将其传递给Boosting分类器。我们训练了一个100个基学习器的分类器,并在测试集上进行了预测。最后,我们输出了模型的准确率。
同样,这也只是一个简单的示例,你可以根据自己的需求进行修改和调整。
相关推荐
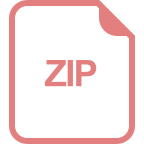
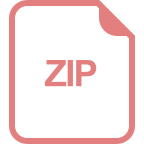










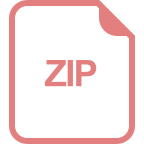
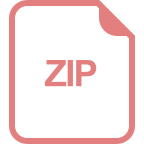