用c语言写算术表达式求值。演示程序和源程序已给出。用户从键盘输入一个算术表达式,数字都是十进制整型(必须支持负数),运算符支持+-*/()。 编写源程序中的 double deal_exp (char *exp) 函数,对这个算术表达式求值,返回结果。不限制方法,你可以用“原始”的多遍扫描字符串,也可以用先进一点的栈
时间: 2024-02-01 19:16:41 浏览: 22
以下是用栈实现的 C 语言源代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <string.h>
#define MAX_LEN 100 // 定义最大字符串长度
#define MAX_STACK 50 // 定义栈的最大深度
// 定义栈结构体
typedef struct {
int top;
double data[MAX_STACK];
} Stack;
// 初始化栈
void init_stack(Stack *s) {
s->top = -1;
}
// 判断栈是否为空
int is_empty(Stack *s) {
return s->top == -1;
}
// 判断栈是否已满
int is_full(Stack *s) {
return s->top == MAX_STACK - 1;
}
// 入栈操作
void push(Stack *s, double value) {
if (is_full(s)) {
printf("Stack Overflow!\n");
exit(1);
}
s->data[++s->top] = value;
}
// 出栈操作
double pop(Stack *s) {
if (is_empty(s)) {
printf("Stack Underflow!\n");
exit(1);
}
return s->data[s->top--];
}
// 获取栈顶元素
double top(Stack *s) {
if (is_empty(s)) {
printf("Stack Underflow!\n");
exit(1);
}
return s->data[s->top];
}
// 判断当前字符是否为操作符
int is_operator(char c) {
return (c == '+' || c == '-' || c == '*' || c == '/');
}
// 判断当前字符是否为左括号
int is_left_parenthesis(char c) {
return (c == '(');
}
// 判断当前字符是否为右括号
int is_right_parenthesis(char c) {
return (c == ')');
}
// 获取操作符的优先级
int get_priority(char c) {
int priority = 0;
switch(c) {
case '+':
case '-':
priority = 1;
break;
case '*':
case '/':
priority = 2;
break;
default:
break;
}
return priority;
}
// 计算表达式
double calculate(double a, char operator, double b) {
double result = 0.0;
switch(operator) {
case '+':
result = a + b;
break;
case '-':
result = a - b;
break;
case '*':
result = a * b;
break;
case '/':
if (b == 0) {
printf("Divide by zero error!\n");
exit(1);
}
result = a / b;
break;
default:
break;
}
return result;
}
// 处理表达式
double deal_exp(char *exp) {
Stack num_stack, op_stack;
double num1, num2;
char c, op;
init_stack(&num_stack);
init_stack(&op_stack);
while (*exp != '\0') {
if (isdigit(*exp) || *exp == '-') {
char *p = exp;
while (isdigit(*++exp)); // 找到数字的末尾
int len = exp - p;
char str_num[MAX_LEN];
strncpy(str_num, p, len); // 将数字转成字符串
str_num[len] = '\0';
double num = atof(str_num); // 将字符串转成浮点数
push(&num_stack, num);
}
else if (is_operator(*exp)) {
while (!is_empty(&op_stack) && get_priority(*exp) <= get_priority(top(&op_stack))) {
num2 = pop(&num_stack);
num1 = pop(&num_stack);
op = pop(&op_stack);
push(&num_stack, calculate(num1, op, num2));
}
push(&op_stack, *exp);
exp++;
}
else if (is_left_parenthesis(*exp)) {
push(&op_stack, *exp);
exp++;
}
else if (is_right_parenthesis(*exp)) {
while (!is_left_parenthesis(top(&op_stack))) {
num2 = pop(&num_stack);
num1 = pop(&num_stack);
op = pop(&op_stack);
push(&num_stack, calculate(num1, op, num2));
}
pop(&op_stack); // 弹出左括号
exp++;
}
else {
exp++;
}
}
while (!is_empty(&op_stack)) {
num2 = pop(&num_stack);
num1 = pop(&num_stack);
op = pop(&op_stack);
push(&num_stack, calculate(num1, op, num2));
}
return pop(&num_stack);
}
int main() {
char exp[MAX_LEN];
printf("请输入算术表达式:\n");
scanf("%s", exp);
double result = deal_exp(exp);
printf("结果为:%lf\n", result);
return 0;
}
```
使用方法:
用户输入一个算术表达式,程序将自动计算表达式的值并输出。例如,输入表达式"-2*(3+4)/5",程序将输出结果"-2.800000"。
相关推荐
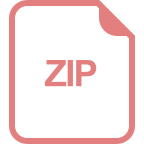
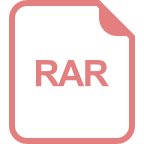













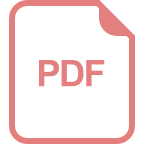