用c语言写算术表达式求值。演示程序和源程序已给出。用户从键盘输入一个算术表达式,数字都是十进制整型(必须支持负数),运算符支持+-*/还有()。 编写源程序中的 double deal_exp (char *exp) 函数,对这个算术表达式求值,返回结果。要求用两个栈,一个储存数字,一个储存运算符
时间: 2024-02-03 20:11:27 浏览: 107
以下是实现算术表达式求值的C语言程序,其中使用了两个栈,一个存储数字,一个存储运算符。
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAX_STACK_SIZE 100
typedef struct {
int top;
int stack[MAX_STACK_SIZE];
} NumStack;
typedef struct {
int top;
char stack[MAX_STACK_SIZE];
} OpStack;
void num_push(NumStack *s, int num) {
if (s->top == MAX_STACK_SIZE - 1) {
printf("Error: number stack overflow.\n");
exit(1);
}
s->stack[++(s->top)] = num;
}
int num_pop(NumStack *s) {
if (s->top == -1) {
printf("Error: number stack underflow.\n");
exit(1);
}
return s->stack[(s->top)--];
}
void op_push(OpStack *s, char op) {
if (s->top == MAX_STACK_SIZE - 1) {
printf("Error: operator stack overflow.\n");
exit(1);
}
s->stack[++(s->top)] = op;
}
char op_pop(OpStack *s) {
if (s->top == -1) {
printf("Error: operator stack underflow.\n");
exit(1);
}
return s->stack[(s->top)--];
}
int priority(char op) {
switch (op) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
case '(':
return 0;
default:
printf("Error: invalid operator '%c'.\n", op);
exit(1);
}
}
double deal_exp(char *exp) {
NumStack num_stack;
OpStack op_stack;
num_stack.top = -1;
op_stack.top = -1;
char *p = exp;
while (*p) {
if (isspace(*p)) {
p++;
} else if (isdigit(*p) || *p == '-') {
int num = 0;
int sign = 1;
if (*p == '-') {
sign = -1;
p++;
}
while (isdigit(*p)) {
num = num * 10 + (*p - '0');
p++;
}
num_push(&num_stack, sign * num);
} else if (*p == '+' || *p == '-' || *p == '*' || *p == '/') {
while (op_stack.top >= 0 && priority(op_stack.stack[op_stack.top]) >= priority(*p)) {
char op = op_pop(&op_stack);
int num2 = num_pop(&num_stack);
int num1 = num_pop(&num_stack);
switch (op) {
case '+':
num_push(&num_stack, num1 + num2);
break;
case '-':
num_push(&num_stack, num1 - num2);
break;
case '*':
num_push(&num_stack, num1 * num2);
break;
case '/':
num_push(&num_stack, num1 / num2);
break;
}
}
op_push(&op_stack, *p);
p++;
} else if (*p == '(') {
op_push(&op_stack, *p);
p++;
} else if (*p == ')') {
while (op_stack.top >= 0 && op_stack.stack[op_stack.top] != '(') {
char op = op_pop(&op_stack);
int num2 = num_pop(&num_stack);
int num1 = num_pop(&num_stack);
switch (op) {
case '+':
num_push(&num_stack, num1 + num2);
break;
case '-':
num_push(&num_stack, num1 - num2);
break;
case '*':
num_push(&num_stack, num1 * num2);
break;
case '/':
num_push(&num_stack, num1 / num2);
break;
}
}
if (op_stack.top == -1) {
printf("Error: unmatched ')'.\n");
exit(1);
}
op_pop(&op_stack);
p++;
} else {
printf("Error: invalid character '%c'.\n", *p);
exit(1);
}
}
while (op_stack.top >= 0) {
char op = op_pop(&op_stack);
int num2 = num_pop(&num_stack);
int num1 = num_pop(&num_stack);
switch (op) {
case '+':
num_push(&num_stack, num1 + num2);
break;
case '-':
num_push(&num_stack, num1 - num2);
break;
case '*':
num_push(&num_stack, num1 * num2);
break;
case '/':
num_push(&num_stack, num1 / num2);
break;
}
}
if (num_stack.top != 0) {
printf("Error: invalid expression.\n");
exit(1);
}
return (double) num_stack.stack[0];
}
int main() {
char exp[MAX_STACK_SIZE];
printf("请输入算术表达式:\n");
fgets(exp, MAX_STACK_SIZE, stdin);
printf("结果是:%.2f\n", deal_exp(exp));
return 0;
}
```
在该程序中,`deal_exp` 函数接受一个算术表达式字符串,使用两个栈对其进行求值,并返回结果。其中 `NumStack` 和 `OpStack` 分别表示数字栈和运算符栈。`num_push` 和 `num_pop` 函数分别表示向数字栈中压入数字和弹出数字。`op_push` 和 `op_pop` 函数分别表示向运算符栈中压入运算符和弹出运算符。`priority` 函数接受一个运算符,返回其优先级。在 `deal_exp` 函数中,通过遍历整个算术表达式字符串,依次处理每个字符,如果遇到数字,则将其转换成整型并压入数字栈中;如果遇到运算符,则将其与运算符栈顶的运算符比较优先级,如果栈顶运算符的优先级不低于当前运算符,则将栈顶运算符弹出,并从数字栈中弹出两个数字进行计算,将计算结果压入数字栈中,然后将当前运算符压入运算符栈中;如果遇到左括号,则将其压入运算符栈中;如果遇到右括号,则将运算符栈顶的运算符弹出,并从数字栈中弹出两个数字进行计算,将计算结果压入数字栈中,直到遇到左括号为止;如果遇到其他字符,则提示输入有误。最后,如果数字栈中只剩下一个数字,则返回该数字,否则提示表达式有误。
阅读全文
相关推荐




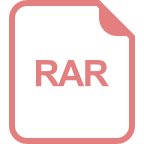
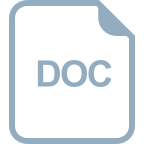
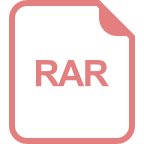
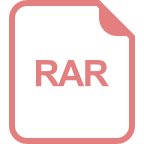
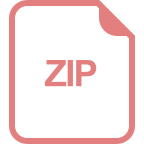
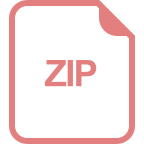
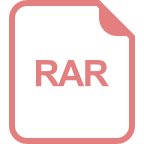
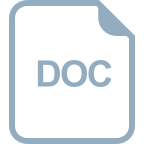
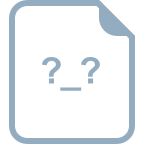
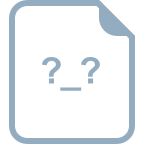
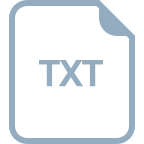
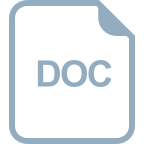