thymeleaf图书管理系统
时间: 2023-12-26 21:05:23 浏览: 26
Thymeleaf是一种用于构建Java应用程序的现代服务器端Java模板引擎。它可以与Spring框架无缝集成,用于生成动态的HTML页面。下面是一个使用Thymeleaf构建图书管理系统的示例:
1. 创建一个Spring Boot项目,并添加Thymeleaf和Spring Boot Web依赖。
2. 在项目的resources/templates目录下创建以下页面模板:
index.html:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>图书管理系统</title>
</head>
<body>
<h1>图书列表</h1>
<table>
<tr>
<th>书名</th>
<th>作者</th>
<th>出版社</th>
<th>图书编号</th>
<th>图书类型</th>
<th>价格</th>
</tr>
<tr th:each="book : ${books}">
<td th:text="${book.title}"></td>
<td th:text="${book.author}"></td>
<td th:text="${book.publisher}"></td>
<td th:text="${book.bookId}"></td>
<td th:text="${book.type}"></td>
<td th:text="${book.price}"></td>
</tr>
</table>
</body>
</html>
```
new_book.html:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>添加图书</title>
</head>
<body>
<h1>添加图书</h1>
<form action="/books" method="post">
<label for="title">书名:</label>
<input type="text" id="title" name="title"><br><br>
<label for="author">作者:</label>
<input type="text" id="author" name="author"><br><br>
<label for="publisher">出版社:</label>
<input type="text" id="publisher" name="publisher"><br><br>
<label for="bookId">图书编号:</label>
<input type="text" id="bookId" name="bookId"><br><br>
<label for="type">图书类型:</label>
<input type="text" id="type" name="type"><br><br>
<label for="price">价格:</label>
<input type="text" id="price" name="price"><br><br>
<input type="submit" value="添加">
</form>
</body>
</html>
```
update_book.html:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>更新图书</title>
</head>
<body>
<h1>更新图书</h1>
<form action="/books/{id}" method="post">
<input type="hidden" name="_method" value="put">
<label for="title">书名:</label>
<input type="text" id="title" name="title" th:value="${book.title}"><br><br>
<label for="author">作者:</label>
<input type="text" id="author" name="author" th:value="${book.author}"><br><br>
<label for="publisher">出版社:</label>
<input type="text" id="publisher" name="publisher" th:value="${book.publisher}"><br><br>
<label for="bookId">图书编号:</label>
<input type="text" id="bookId" name="bookId" th:value="${book.bookId}"><br><br>
<label for="type">图书类型:</label>
<input type="text" id="type" name="type" th:value="${book.type}"><br><br>
<label for="price">价格:</label>
<input type="text" id="price" name="price" th:value="${book.price}"><br><br>
<input type="submit" value="更新">
</form>
</body>
</html>
```
search.html:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>查询图书</title>
</head>
<body>
<h1>查询图书</h1>
<form action="/books/search" method="get">
<label for="keyword">关键字:</label>
<input type="text" id="keyword" name="keyword"><br><br>
<input type="submit" value="查询">
</form>
</body>
</html>
```
3. 创建一个Book类来表示图书信息,并在控制器中实现增删改查的功能。
```java
@Controller
public class BookController {
private List<Book> books = new ArrayList<>();
@GetMapping("/books")
public String getAllBooks(Model model) {
model.addAttribute("books", books);
return "index";
}
@GetMapping("/books/new")
public String showNewBookForm(Model model) {
model.addAttribute("book", new Book());
return "new_book";
}
@PostMapping("/books")
public String addBook(@ModelAttribute Book book) {
books.add(book);
return "redirect:/books";
}
@GetMapping("/books/{id}/edit")
public String showUpdateBookForm(@PathVariable int id, Model model) {
Book book = books.get(id);
model.addAttribute("book", book);
return "update_book";
}
@PutMapping("/books/{id}")
public String updateBook(@PathVariable int id, @ModelAttribute Book updatedBook) {
Book book = books.get(id);
book.setTitle(updatedBook.getTitle());
book.setAuthor(updatedBook.getAuthor());
book.setPublisher(updatedBook.getPublisher());
book.setBookId(updatedBook.getBookId());
book.setType(updatedBook.getType());
book.setPrice(updatedBook.getPrice());
return "redirect:/books";
}
@GetMapping("/books/search")
public String searchBooks(@RequestParam String keyword, Model model) {
List<Book> searchResults = new ArrayList<>();
for (Book book : books) {
if (book.getTitle().contains(keyword) || book.getAuthor().contains(keyword)) {
searchResults.add(book);
}
}
model.addAttribute("books", searchResults);
return "index";
}
// 其他方法:删除图书等
}
```
以上是一个简单的使用Thymeleaf构建的图书管理系统示例。你可以根据自己的需求进行扩展和修改。
相关推荐
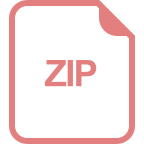
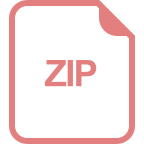














