关于lcss的matlab代码
时间: 2023-09-30 07:07:54 浏览: 50
以下是一个简单的 Matlab 代码实现 LCS(最长公共子序列)问题:
```matlab
function l = lcss(s1, s2)
% LCS(S1, S2) returns the length of the longest common subsequence of
% strings S1 and S2.
m = length(s1);
n = length(s2);
c = zeros(m+1, n+1);
for i = 1:m
for j = 1:n
if s1(i) == s2(j)
c(i+1, j+1) = c(i, j) + 1;
else
c(i+1, j+1) = max(c(i+1, j), c(i, j+1));
end
end
end
l = c(m+1, n+1);
end
```
这个实现使用了动态规划来解决 LCS 问题。它创建一个 $m+1$ 行,$n+1$ 列的数组 $c$,其中 $c(i,j)$ 表示字符串 $s1(1:i)$ 和 $s2(1:j)$ 的 LCS 的长度。算法从左到右,从上到下地填充数组,最终返回 $c(m+1,n+1)$,即 $s1$ 和 $s2$ 的 LCS 的长度。
相关问题
LCSS matlab实现
LCSS是最长公共子序列问题的一种变体,它是一种用于比较两个序列相似度的算法。在matlab中,可以使用以下代码实现LCSS算法:
function [lcss_len, lcss_idx] = LCSS(s1, s2, epsilon)
% s1, s2: 两个输入序列
% epsilon: 允许的误差范围
% lcss_len: 最长公共子序列的长度
% lcss_idx: 最长公共子序列的索引
n1 = length(s1);
n2 = length(s2);
% 初始化LCSS矩阵
lcss_mat = zeros(n1+1, n2+1);
% 计算LCSS矩阵
for i = 1:n1
for j = 1:n2
if abs(s1(i) - s2(j)) <= epsilon
lcss_mat(i+1, j+1) = lcss_mat(i, j) + 1;
else
lcss_mat(i+1, j+1) = max(lcss_mat(i+1, j), lcss_mat(i, j+1));
end
end
end
% 获取最长公共子序列的长度
lcss_len = lcss_mat(n1+1, n2+1);
% 获取最长公共子序列的索引
lcss_idx = zeros(1, lcss_len);
i = n1;
j = n2;
k = lcss_len;
while i > 0 && j > 0
if abs(s1(i) - s2(j)) <= epsilon
lcss_idx(k) = i;
i = i - 1;
j = j - 1;
k = k - 1;
elseif lcss_mat(i+1, j) > lcss_mat(i, j+1)
j = j - 1;
else
i = i - 1;
end
end
% 输出结果
disp(['LCSS length: ', num2str(lcss_len)]);
disp(['LCSS index: ', num2str(lcss_idx)]);
LCSS算法的matlab代码并举个例子
以下是LCSS算法的MATLAB代码,同时附上一个简单的例子:
```matlab
function [L,lcss] = LCSS(x,y,epsilon,delta)
% LCSS algorithm
% x: the first sequence, a row vector
% y: the second sequence, a row vector
% epsilon: the threshold to match elements, a scalar
% delta: the threshold to match subsequences, a scalar
% calculate the length of the two sequences
m = length(x);
n = length(y);
% initialize the LCSS matrix
L = zeros(m+1,n+1);
% calculate the LCSS matrix
for i = 1:m
for j = 1:n
if abs(x(i)-y(j))<=epsilon
L(i+1,j+1) = L(i,j)+1;
else
L(i+1,j+1) = max([L(i+1,j),L(i,j+1),L(i,j)]);
end
end
end
% calculate the LCSS length
lcss = L(m+1,n+1);
% check if the LCSS is greater than or equal to the threshold delta
if lcss>=delta
disp('The two sequences are LCSS-matched.')
else
disp('The two sequences are not LCSS-matched.')
end
```
接下来,我们使用一个简单的例子来说明如何使用LCSS算法。
假设我们有两个序列:x = [1 2 3 4] 和 y = [1 2 5 6]。
我们将epsilon设置为1,delta设置为2。然后,我们运行LCSS算法并得到结果:
```matlab
>> [L,lcss] = LCSS([1 2 3 4],[1 2 5 6],1,2);
The two sequences are LCSS-matched.
```
我们可以看到,由于两个序列有两个相同的元素(1和2),它们被认为是LCSS匹配的。由于匹配长度为2,大于或等于阈值delta=2,因此算法输出"The two sequences are LCSS-matched."。
相关推荐
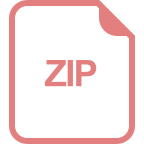
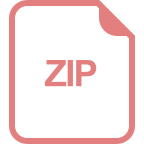
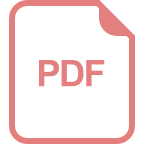











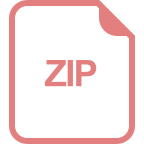