JavaScript获取指定年份的2月份的天数
时间: 2024-11-22 16:35:27 浏览: 13
在JavaScript中,你可以通过Date对象的getMonth()和getDate()方法以及一些额外的逻辑来获取指定年份二月的天数。由于闰年的规则(二月有29天),需要判断当前年份是否是闰年:
```javascript
function getDaysInFebruary(year) {
// 判断是否是闰年
function isLeapYear(year) {
return (year % 4 === 0 && year % 100 !== 0) || (year % 400 === 0);
}
const date = new Date(year, 1); // 月份从0开始计数,所以2月是1
date.setMonth(1, 1); // 设置日期为1日,因为getMonth()返回的是剩余的天数
if (isLeapYear(year)) {
return date.getDate(); // 如果是闰年,二月就有29天
} else {
return 28; // 非闰年,二月有28天
}
}
// 使用示例
const daysInFeb2023 = getDaysInFebruary(2023);
console.log(daysInFeb2023); // 输出2023年2月的天数
相关问题
JavaScript闭包获取指定年份的2月份的天数
JavaScript中的闭包是一种特殊的作用域特性,它允许访问在其外部函数作用域内的变量,即使这个外部函数已经返回了。关于获取指定年份的二月天数,我们可以创建一个闭包函数来处理闰年和平年的规则。
下面是一个简单的例子:
```javascript
function getDaysInFebruary(year) {
// 使用立即执行函数表达式创建闭包
return (function(isLeapYear) {
// 内部函数判断是否为闰年
function isLeap() {
return (year % 4 === 0 && year % 100 !== 0) || (year % 400 === 0);
}
// 根据闰年规则计算二月天数
var days = isLeap() ? 29 : 28;
return days;
})(year % 4 === 0); // 将判断闰年的条件作为实参传递给内部函数
}
// 调用函数并传入特定年份
var daysInFeb2023 = getDaysInFebruary(2023);
console.log(daysInFeb2023); // 输出相应年份的二月天数
JavaScript编写函数获取指定年份的2月份的天数?
在JavaScript中,你可以编写一个函数来获取给定年份二月的天数,因为闰年的二月有29天,非闰年的二月则有28天。这里有一个简单的函数示例:
```javascript
function getDaysInFebruary(year) {
// 判断是否为闰年
function isLeapYear() {
return (year % 4 === 0 && year % 100 !== 0) || (year % 400 === 0);
}
const days = isLeapYear() ? 29 : 28;
return days;
}
// 使用示例
let year = 2023; // 或者你需要查询的任何年份
const daysInFeb = getDaysInFebruary(year);
console.log(`年份 ${year} 的二月份有 ${daysInFeb} 天.`);
```
这个`getDaysInFebruary`函数首先检查输入的年份是否为闰年,然后返回相应的天数。
阅读全文
相关推荐
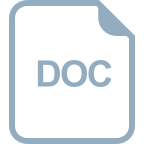
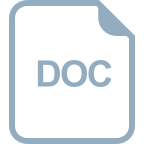
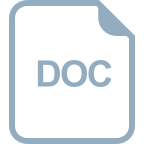



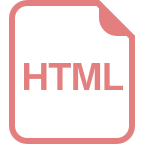
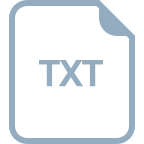
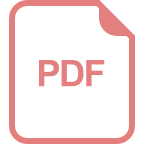
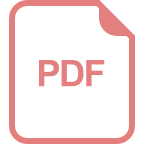






