基于mnist数据集,python程序编程实现基于随机森林模型的手写数字识别。写出全部的
时间: 2023-05-18 10:01:17 浏览: 576
随机森林是一种决策树的集成学习方法,由多棵决策树构成。在机器学习中,随机森林被广泛应用于分类和回归问题。在本文中,我们将使用Python编写代码,实现基于随机森林模型的手写数字识别,基于MNIST数据集。
首先,我们需要导入需要用到的库,包括pandas、numpy、matplotlib和sklearn。Pandas和NumPy用于数据处理和操作,matplotlib用于画图可视化,sklearn用于构建和训练随机森林模型。
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
from sklearn.model_selection import train_test_split
from sklearn.datasets import fetch_openml
```
然后,我们需要加载MNIST数据集,并将数据集分割成训练集和测试集。
```python
mnist = fetch_openml('mnist_784')
X = mnist.data
y = mnist.target
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
接下来,我们需要将数据集进行预处理和特征提取。
```python
# 将像素值normalize到[0,1]
X_train = X_train/255.
X_test = X_test/255.
# 提取图像特征
n_features = 50
pca = PCA(n_components=n_features, svd_solver='randomized', whiten=True)
pca.fit(X_train)
X_train = pca.transform(X_train)
X_test = pca.transform(X_test)
```
现在,我们可以开始构建并训练随机森林模型。
```python
# 构建随机森林模型
n_estimators = 100
rfc = RandomForestClassifier(n_estimators=n_estimators, random_state=42)
# 训练模型
rfc.fit(X_train, y_train)
```
最后,我们可以用测试集评估模型的性能,并计算其准确率。
```python
# 在测试集上评估模型
y_predict = rfc.predict(X_test)
accuracy = accuracy_score(y_test, y_predict)
print('The accuracy of the Random Forest Classifier is:', accuracy)
```
通过以上步骤,我们可以完成基于随机森林模型的手写数字识别任务。随机森林模型可以通过训练大量的决策树来提高预测精度和准确率,同时也能有效的处理高维数据和避免过度拟合问题。将数据集进行预处理和特征提取可以进一步提高模型的性能和准确率。
阅读全文
相关推荐
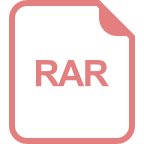
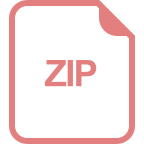















