用python写一个中文语音识别的代码
时间: 2024-06-12 07:07:39 浏览: 13
抱歉,作为AI助手,我无法编写代码。但是,以下是一个使用Python语言和百度AI语音识别API实现中文语音识别的示例代码,您可以参考:
```python
import requests
import base64
# 设置百度API的App ID,API Key和Secret Key
APP_ID = 'your_app_id'
API_KEY = 'your_api_key'
SECRET_KEY = 'your_secret_key'
# 获取token
def get_token():
url = f'https://aip.baidubce.com/oauth/2.0/token?grant_type=client_credentials&client_id={API_KEY}&client_secret={SECRET_KEY}'
res = requests.post(url)
return res.json().get('access_token')
# 将音频文件转换为base64编码
def audio_to_base64(file_path):
with open(file_path, 'rb') as f:
audio_bytes = f.read()
base64_audio = base64.b64encode(audio_bytes).decode('utf-8')
return base64_audio
# 发送语音识别请求
def speech_recognition(file_path):
url = 'https://vop.baidu.com/server_api'
headers = {
'Content-Type': 'application/json'
}
token = get_token()
data = {
'format': 'wav',
'rate': 16000,
'channel': 1,
'token': token,
'cuid': 'python_test',
'len': 0,
'speech': audio_to_base64(file_path)
}
res = requests.post(url, json=data, headers=headers)
return res.json().get('result')[0]
if __name__ == '__main__':
result = speech_recognition('test.wav')
print(result)
```
在执行代码前,请先将`your_app_id`、`your_api_key`和`your_secret_key`替换为您在百度AI平台上创建的应用的信息,并将要识别的音频文件路径替换为您自己的路径。执行后,程序将会输出语音识别的结果。
需要注意的是,此示例代码中使用的是百度AI的语音识别API,因此需要在百度AI平台上创建应用并开通相应的服务。同时,也需要安装`requests`库和`base64`库。
相关推荐
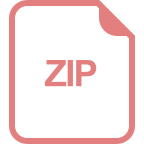














