java 曲线计算_如何计算曲线下的部分面积(AUC)
时间: 2024-03-02 15:52:26 浏览: 108
要计算曲线下的部分面积(AUC),可以按照以下步骤进行:
1. 将曲线分成多个小曲线段,每个小曲线段可以看成一个梯形,其上底和下底对应于两个相邻的点的纵坐标,高对应于两个相邻点的横坐标之差。计算每个小梯形的面积。
2. 将所有小梯形的面积相加,得到曲线下的总面积。
3. 如果曲线是一个封闭的曲线,需要减去曲线上方的面积,即曲线内部的面积,才能得到曲线下的部分面积。
Java中可以使用数值积分的方法来计算曲线下的面积,例如梯形法、辛普森法等。这些方法可以在Java中的数学库中找到相应的实现。举个例子,使用梯形法计算曲线下的面积的代码如下:
```java
public double calculateAUC(double[] x, double[] y) {
double area = 0.0;
for (int i = 1; i < x.length; i++) {
double h = x[i] - x[i-1];
double avg = (y[i] + y[i-1]) / 2.0;
area += h * avg;
}
return area;
}
```
其中,x和y分别是曲线上各点的横坐标和纵坐标,使用for循环依次计算每个小梯形的面积,并将面积累加到总面积中。最后返回总面积即可。
相关问题
请用c语言或java实现
好的,以下是使用 C 语言和标准库函数实现 ROC 曲线的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define MAX_SAMPLE_NUM 10
// 样本的真实标签
int y_true[MAX_SAMPLE_NUM] = {1, 0, 1, 1, 0, 1, 0, 0, 0, 1};
// 样本的预测概率值
double y_score[MAX_SAMPLE_NUM] = {0.95, 0.85, 0.78, 0.66, 0.6, 0.55, 0.53, 0.52, 0.51, 0.4};
// 计算 ROC 曲线的假正率、真正率和阈值
void roc_curve(double *fpr, double *tpr, double *thresholds, int n_samples) {
int n_pos = 0, n_neg = 0;
for (int i = 0; i < n_samples; i++) {
if (y_true[i] == 1) {
n_pos++;
} else {
n_neg++;
}
}
int tp = 0, fp = 0;
double prev_threshold = -INFINITY;
for (int i = 0; i < n_samples; i++) {
if (y_score[i] != prev_threshold) {
fpr[tp + fp] = (double) fp / n_neg;
tpr[tp + fp] = (double) tp / n_pos;
thresholds[tp + fp] = y_score[i];
prev_threshold = y_score[i];
}
if (y_true[i] == 1) {
tp++;
} else {
fp++;
}
}
fpr[tp + fp] = (double) fp / n_neg;
tpr[tp + fp] = (double) tp / n_pos;
thresholds[tp + fp] = 1.0;
}
// 计算 ROC 曲线的面积 AUC
double auc(double *fpr, double *tpr, int n_samples) {
double auc = 0.0;
for (int i = 1; i < n_samples; i++) {
auc += (tpr[i] + tpr[i - 1]) * (fpr[i - 1] - fpr[i]) / 2.0;
}
return auc;
}
// 绘制 ROC 曲线
void plot_roc_curve(double *fpr, double *tpr, int n_samples) {
FILE *gnuplot = popen("gnuplot -persistent", "w");
fprintf(gnuplot, "set terminal pngcairo size 640,480 enhanced font 'Verdana,10'\n");
fprintf(gnuplot, "set output 'roc_curve.png'\n");
fprintf(gnuplot, "set xlabel 'False Positive Rate'\n");
fprintf(gnuplot, "set ylabel 'True Positive Rate'\n");
fprintf(gnuplot, "set title 'ROC Curve'\n");
fprintf(gnuplot, "set grid\n");
fprintf(gnuplot, "plot '-' with lines title 'ROC curve'\n");
for (int i = 0; i < n_samples; i++) {
fprintf(gnuplot, "%f %f\n", fpr[i], tpr[i]);
}
fprintf(gnuplot, "1.0 1.0\n");
fprintf(gnuplot, "e\n");
fflush(gnuplot);
}
int main() {
double fpr[MAX_SAMPLE_NUM + 1];
double tpr[MAX_SAMPLE_NUM + 1];
double thresholds[MAX_SAMPLE_NUM + 1];
roc_curve(fpr, tpr, thresholds, MAX_SAMPLE_NUM);
double roc_auc = auc(fpr, tpr, MAX_SAMPLE_NUM + 1);
printf("AUC: %f\n", roc_auc);
plot_roc_curve(fpr, tpr, MAX_SAMPLE_NUM + 1);
return 0;
}
```
代码中,我们使用了 `math.h` 头文件中的 `INFINITY` 宏来表示无穷大。首先,我们定义了样本的真实标签和预测概率值,并使用 `roc_curve` 函数计算了 ROC 曲线的假正率、真正率和阈值。其中,我们使用了两个计数器 `n_pos` 和 `n_neg` 分别统计正样本和负样本的数量,遍历样本时计算了真正率、假正率和阈值,并将结果存储在 `fpr`、`tpr` 和 `thresholds` 数组中。
然后,我们使用 `auc` 函数计算 ROC 曲线的面积 AUC,并使用 `plot_roc_curve` 函数绘制 ROC 曲线。其中,我们使用标准库函数 `popen` 打开了一个 GNUPlot 进程,并向其发送绘图命令,最终将绘制结果保存为 PNG 图像文件。你可以在运行程序后查看当前目录下的 `roc_curve.png` 图像文件。
值得注意的是,为了绘制 ROC 曲线,我们需要使用第三方工具库,比如 GNUPlot。因此,你需要预先安装并配置好这些工具库才能成功绘制 ROC 曲线。
阅读全文
相关推荐
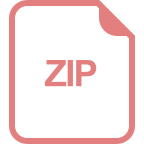
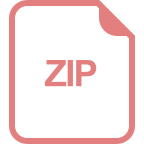
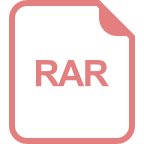
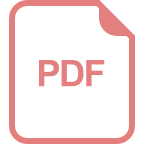
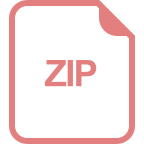
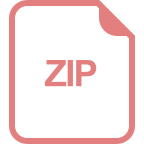
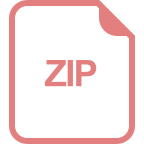
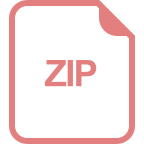
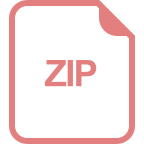
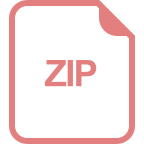
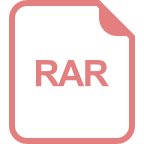
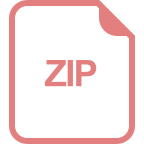
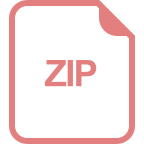
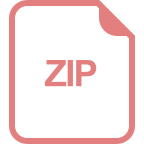
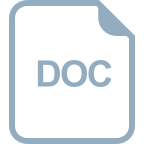
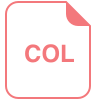
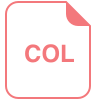
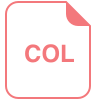