请写一段AirSim无人机在三维空间中实现A*+DWA算法的代码。
时间: 2024-10-03 16:04:16 浏览: 41
在AirSim(Microsoft的开源飞行模拟平台)中,要实现A* + DWA(动态窗口避障路径规划算法)算法,你需要首先安装AirSim库并了解其Python API。这里是一个简化的示例代码片段,展示了如何在一个基本框架内集成这两个导航算法:
```python
import numpy as np
from airsim.types import Pose, Vector3r
from airsim.utils import distance
class AstarDwaPlanner:
def __init__(self, start_pose, goal_pose):
self.start_pose = start_pose
self.goal_pose = goal_pose
self.map_data = load_map_data() # 假设已加载地图数据
self.astar_graph = build_astar_graph(map_data) # 构建A*图
self.dwa_params = DWAParams() # 设置DWA参数
def plan_path(self):
astar_path = self.astar_search(start_pose, goal_pose)
if astar_path is not None:
dwa_path = self.execute_dwa(astar_path)
return dwa_path
else:
print("No path found from start to goal")
return []
def astar_search(self, start, goal):
# 使用A*搜索算法找到最短路径
pass # 实现细节取决于AirSim的API和自定义的A*算法
def execute_dwa(self, path):
current_pose = start_pose
waypoints = [pose.position for pose in path]
while current_pose != goal_pose:
next_waypoint = self.select_next_waypoint(waypoints, current_pose)
current_pose = self.move_robot_to_next_waypoint(current_pose, next_waypoint)
return path
def select_next_waypoint(self, waypoints, current_pose):
# 根据DWA参数计算下一个安全目标点
# 这里需要考虑速度、障碍物避免等
pass
def move_robot_to_next_waypoint(self, current_pose, next_waypoint):
# 发送移动命令到AirSim控制飞机到下一目标点
client = MultirotorClient()
client.move_by_local_position_async(Vector3r(next_waypoint.x_val, next_waypoint.y_val, current_pose.z_val), True)
return client.get_current_position().position
# ... 定义DWAParams类和其他必要的辅助函数
```
注意,这只是一个基础的模板,并未包含完整的A*搜索和DWA路径跟踪的所有细节,例如代价函数的选择、启发式函数的实现、局部路径调整以及处理AirSim实时反馈等。实际应用中,你还需要根据AirSim的具体文档和功能进行相应的修改。
阅读全文
相关推荐
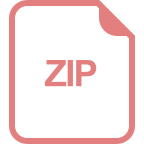
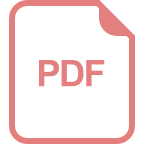
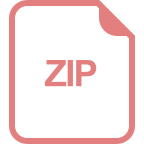

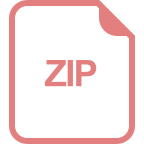











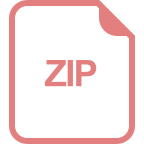