学生的记录由姓名和成绩组成且在主函数中通过结构体数组初始化,无需输入。请编写函数实现把输入的指定分数范围内的学生通过结构体指针保存数据并输出。
时间: 2023-11-27 21:53:34 浏览: 35
以下是一个示例代码,其中包括了结构体定义、初始化结构体数组和实现功能的函数。
```c
#include <stdio.h>
#define NUM_STUDENTS 5
struct student {
char name[20];
int score;
};
void print_students_within_range(struct student *students, int num_students, int min_score, int max_score) {
printf("Students within range of %d to %d:\n", min_score, max_score);
for (int i = 0; i < num_students; i++) {
if (students[i].score >= min_score && students[i].score <= max_score) {
printf("%s: %d\n", students[i].name, students[i].score);
}
}
}
int main() {
struct student students[NUM_STUDENTS] = {
{"Alice", 85},
{"Bob", 73},
{"Charlie", 92},
{"David", 68},
{"Eve", 77}
};
int min_score = 75;
int max_score = 90;
print_students_within_range(students, NUM_STUDENTS, min_score, max_score);
return 0;
}
```
在这个示例代码中,我们首先定义了一个结构体 `student`,包括了学生的姓名和分数。然后在 `main` 函数中,我们初始化了一个包含 5 个学生结构体的数组,并设置了最小分数和最大分数的范围。最后,我们调用了 `print_students_within_range` 函数,该函数通过循环遍历每个学生结构体,判断其分数是否在指定范围内,如果是则输出对应的学生姓名和分数。
相关推荐
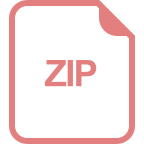
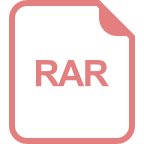
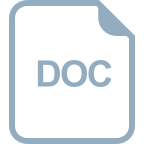















