c语言用结构体数组统计学生成绩
时间: 2024-12-25 19:12:14 浏览: 7
在C语言中,我们可以使用结构体数组来存储学生的成绩信息,比如姓名、学号和分数。首先,我们需要定义一个包含这些属性的结构体:
```c
struct Student {
char name[50]; // 学生姓名
int id; // 学号
float score; // 成绩
};
```
然后创建一个结构体数组,用于存放多个学生的信息:
```c
#define NUM_OF_STUDENTS 10 // 假设有10个学生
struct Student students[NUM_OF_STUDENTS];
```
为了统计生成绩,我们可以在程序的不同部分编写函数,如计算平均分、最高分和最低分:
```c
void calculate_average(struct Student* students, int num_students) {
float total = 0;
for (int i = 0; i < num_students; i++) {
total += students[i].score;
}
printf("平均分: %.2f\n", total / num_students);
}
float get_max_score(struct Student* students, int num_students) {
float max_score = students[0].score;
for (int i = 1; i < num_students; i++) {
if (students[i].score > max_score) {
max_score = students[i].score;
}
}
return max_score;
}
float get_min_score(struct Student* students, int num_students) {
float min_score = students[0].score;
for (int i = 1; i < num_students; i++) {
if (students[i].score < min_score) {
min_score = students[i].score;
}
}
return min_score;
}
```
最后,在主函数中初始化学生数据并调用这些函数:
```c
int main() {
// 初始化学生数组...
// 计算平均分
calculate_average(students, NUM_OF_STUDENTS);
// 打印最高分
printf("最高分: %.2f\n", get_max_score(students, NUM_OF_STUDENTS));
// 打印最低分
printf("最低分: %.2f\n", get_min_score(students, NUM_OF_STUDENTS));
return 0;
}
```
阅读全文
相关推荐
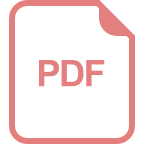
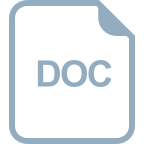
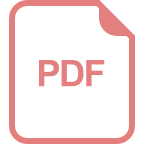
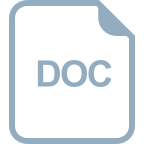





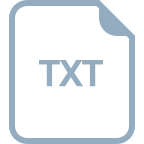
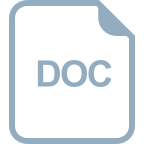







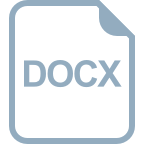