用c语音写一段代码:定义包含5个元素的数组,输入数据,然后再输入一个数,在数值中查询是否存在这个数,存在则删除这个数,输出数组。
时间: 2024-10-22 08:21:24 浏览: 17
在C语言中,虽然我无法直接提供语音指导,但我可以为你展示如何编写相应的代码片段来完成这个任务。首先,你需要包含一些头文件,然后定义数组,并处理用户输入。以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define SIZE 5
// 定义一个整型数组
int array[SIZE];
void input_data() {
int i;
for (i = 0; i < SIZE; i++) {
printf("Enter element %d: ", i+1);
scanf("%d", &array[i]);
}
}
void delete_element(int target) {
int i, found = 0;
for (i = 0; i < SIZE && !found; i++) {
if (array[i] == target) {
found = 1;
// 使用数组溢出检查防止误操作
memmove(&array[i], &array[i + 1], (SIZE - i - 1) * sizeof(int));
}
}
}
void print_array() {
int i;
for (i = 0; i < SIZE; i++) {
printf("%d ", array[i]);
}
printf("\n");
}
int main() {
input_data();
int search_num;
printf("Enter a number to search and potentially delete: ");
scanf("%d", &search_num);
delete_element(search_num);
print_array();
return 0;
}
```
这段代码首先提示用户输入5个数字到数组里,然后接收一个搜索值。如果找到该值,则从数组中移除它。最后,程序会打印修改后的数组。
注意,这只是一个基础版本,实际应用中可能需要考虑更多边界情况和错误处理。
阅读全文
相关推荐
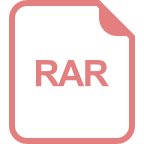
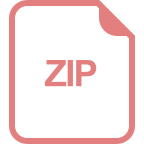
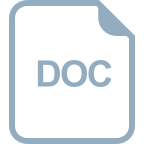
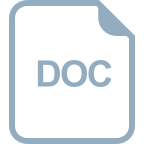
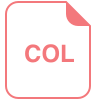
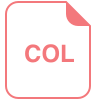
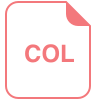
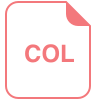
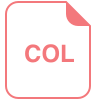
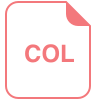
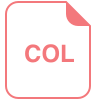
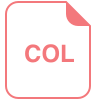
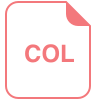
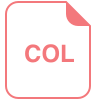
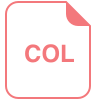
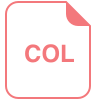
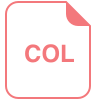
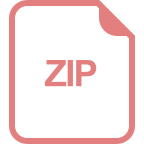