rsa数字签名c++
时间: 2023-12-02 12:40:55 浏览: 68
RSA是一种非对称加密算法,可以用于数字签名。下面是一个使用C++实现RSA数字签名的例子:
```cpp
#include <iostream>
#include <string>
#include <openssl/rsa.h>
#include <openssl/pem.h>
using namespace std;
int main()
{
// 生成RSA密钥对
RSA *keypair = RSA_generate_key(2048, RSA_F4, NULL, NULL);
if (keypair == NULL) {
cout << "Failed to generate RSA key pair." << endl;
return 1;
}
// 获取私钥和公钥
BIO *pri = BIO_new(BIO_s_mem());
BIO *pub = BIO_new(BIO_s_mem());
PEM_write_bio_RSAPrivateKey(pri, keypair, NULL, NULL, 0, NULL, NULL);
PEM_write_bio_RSAPublicKey(pub, keypair);
// 从BIO中读取私钥和公钥
char *pri_key = NULL;
char *pub_key = NULL;
long pri_len = BIO_get_mem_data(pri, &pri_key);
long pub_len = BIO_get_mem_data(pub, &pub_key);
// 签名
string message = "Hello, world!";
unsigned char *sig = new unsigned char[RSA_size(keypair)];
unsigned int sig_len;
RSA_sign(NID_sha256, (const unsigned char *)message.c_str(), message.length(), sig, &sig_len, keypair);
// 验证签名
int result = RSA_verify(NID_sha256, (const unsigned char *)message.c_str(), message.length(), sig, sig_len, keypair);
if (result == 1) {
cout << "Signature verified." << endl;
} else {
cout << "Signature verification failed." << endl;
}
// 输出私钥和公钥
cout << "Private key:" << endl << string(pri_key, pri_len) << endl;
cout << "Public key:" << endl << string(pub_key, pub_len) << endl;
// 释放资源
RSA_free(keypair);
BIO_free_all(pri);
BIO_free_all(pub);
delete[] sig;
return 0;
}
```
相关推荐
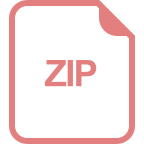
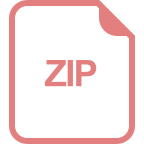














