使用c语言和jpeg库实现将jpeg图片转成NV21格式的yuv数据
时间: 2023-08-29 12:07:42 浏览: 279
要将JPEG图片转换为NV21格式的YUV数据,需要使用libjpeg库解码JPEG图片,然后使用YUV转换算法将RGB数据转换为YUV格式。以下是一个使用C语言和libjpeg库实现此功能的示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <jpeglib.h>
#define YUV420P_PIXEL_SIZE 3
#define RGB_PIXEL_SIZE 3
int main(int argc, char** argv) {
if (argc != 3) {
printf("Usage: jpeg2nv21 <input_file> <output_file>\n");
return 0;
}
char* input_file = argv[1];
char* output_file = argv[2];
// Open input file
FILE* infile = fopen(input_file, "rb");
if (infile == NULL) {
printf("Could not open input file: %s\n", input_file);
return 0;
}
// Initialize libjpeg structures
struct jpeg_decompress_struct cinfo;
struct jpeg_error_mgr jerr;
cinfo.err = jpeg_std_error(&jerr);
jpeg_create_decompress(&cinfo);
jpeg_stdio_src(&cinfo, infile);
// Read JPEG header
jpeg_read_header(&cinfo, TRUE);
// Start decompression
jpeg_start_decompress(&cinfo);
// Allocate memory for RGB buffer
int row_stride = cinfo.output_width * RGB_PIXEL_SIZE;
JSAMPARRAY buffer = (*cinfo.mem->alloc_sarray)
((j_common_ptr) &cinfo, JPOOL_IMAGE, row_stride, 1);
int rgb_size = cinfo.output_width * cinfo.output_height * RGB_PIXEL_SIZE;
unsigned char* rgb_data = (unsigned char*) malloc(rgb_size);
// Read RGB data
int row_index = 0;
while (cinfo.output_scanline < cinfo.output_height) {
jpeg_read_scanlines(&cinfo, buffer, 1);
memcpy(rgb_data + row_index * row_stride, buffer[0], row_stride);
row_index++;
}
// Finish decompression
jpeg_finish_decompress(&cinfo);
jpeg_destroy_decompress(&cinfo);
fclose(infile);
// Convert RGB to NV21
int y_size = cinfo.output_width * cinfo.output_height;
int uv_size = y_size / 2;
unsigned char* nv21_data = (unsigned char*) malloc(y_size + uv_size);
int y_index = 0;
int uv_index = 0;
for (int i = 0; i < rgb_size; i += RGB_PIXEL_SIZE) {
int r = rgb_data[i];
int g = rgb_data[i + 1];
int b = rgb_data[i + 2];
// Convert RGB to YUV
int y = (int) (0.299 * r + 0.587 * g + 0.114 * b);
int u = (int) (-0.169 * r - 0.331 * g + 0.5 * b + 128);
int v = (int) (0.5 * r - 0.419 * g - 0.081 * b + 128);
// Write Y data
nv21_data[y_index] = (unsigned char) y;
y_index++;
// Write UV data
if (i % (2 * RGB_PIXEL_SIZE) == 0) {
nv21_data[y_size + uv_index] = (unsigned char) v;
nv21_data[y_size + uv_size + uv_index] = (unsigned char) u;
uv_index++;
}
}
// Write NV21 data to output file
FILE* outfile = fopen(output_file, "wb");
if (outfile == NULL) {
printf("Could not open output file: %s\n", output_file);
return 0;
}
fwrite(nv21_data, 1, y_size + uv_size, outfile);
fclose(outfile);
// Free memory
free(rgb_data);
free(nv21_data);
return 0;
}
```
注意,这是一个简单的示例程序,可能需要根据具体需求进行修改。在使用此程序时,需要将libjpeg库链接到编译命令中。具体方法可以参考libjpeg库的说明文档。
阅读全文
相关推荐
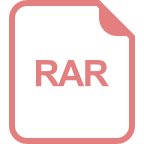
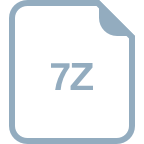
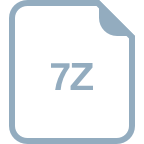
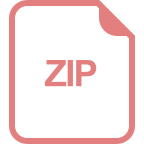
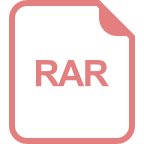
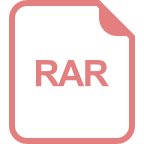
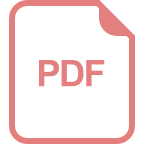
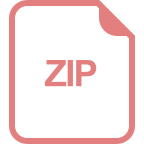
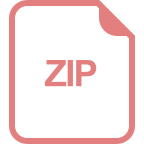
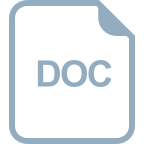
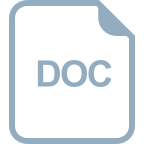
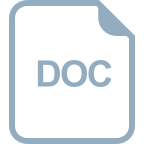
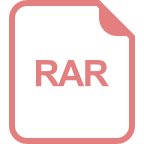
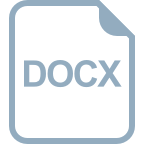