从现有IP数据文件中提取所有的证书并写入cer文件 c语言代码
时间: 2024-03-05 14:51:15 浏览: 90
以下是一个基本的C语言代码示例,用于从现有IP数据文件中提取所有的证书并写入cer文件:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_CERT_LENGTH 8192 // 最大证书长度
int main(int argc, char* argv[])
{
FILE *fp_in, *fp_out;
char *buffer;
char *cert_begin, *cert_end;
int bytes_read, cert_length, total_bytes_read, num_certs_written;
if(argc != 3)
{
printf("Usage: %s [input filename] [output filename]\n", argv[0]);
return 0;
}
fp_in = fopen(argv[1], "rt");
if(fp_in == NULL)
{
printf("Error opening input file: %s\n", argv[1]);
return 0;
}
fp_out = fopen(argv[2], "wb");
if(fp_out == NULL)
{
printf("Error creating output file: %s\n", argv[2]);
fclose(fp_in);
return 0;
}
buffer = (char*)malloc(MAX_CERT_LENGTH);
num_certs_written = 0;
total_bytes_read = 0;
while(!feof(fp_in))
{
bytes_read = fread(buffer, 1, MAX_CERT_LENGTH, fp_in);
total_bytes_read += bytes_read;
cert_begin = buffer;
while((cert_begin = strstr(cert_begin, "-----BEGIN CERTIFICATE-----")) != NULL)
{
cert_end = strstr(cert_begin, "-----END CERTIFICATE-----");
if(cert_end == NULL)
{
printf("Error: could not find end of certificate.\n");
free(buffer);
fclose(fp_in);
fclose(fp_out);
return 0;
}
cert_end += strlen("-----END CERTIFICATE-----");
cert_length = cert_end - cert_begin;
fwrite(cert_begin, 1, cert_length, fp_out);
num_certs_written++;
cert_begin = cert_end;
}
}
printf("Total bytes read: %d\n", total_bytes_read);
printf("Certificates extracted: %d\n", num_certs_written);
free(buffer);
fclose(fp_in);
fclose(fp_out);
return 0;
}
```
该程序的基本流程如下:
1. 打开输入文件和输出文件。
2. 从输入文件中读取数据,直到到达文件末尾。
3. 在读取的数据中查找每个证书的开始和结束标记。
4. 将每个证书写入输出文件。
5. 关闭文件并释放内存。
注意,该程序仅提供了基本的证书提取功能,可能需要根据特定数据文件的格式进行修改。此外,该程序还假定证书的最大长度为8192,如果证书超过该长度,可能会导致程序崩溃或无法正确提取证书。
阅读全文
相关推荐
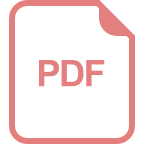
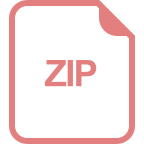




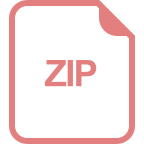
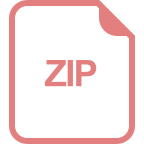
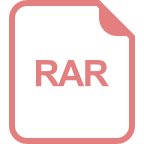
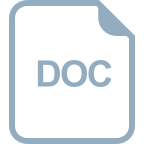







