从现有文件中提取其IP数据包中的证书,并写入cer文件 c语言完整代码
时间: 2024-02-27 21:51:38 浏览: 127
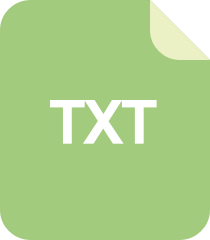
SSL中各证书的转换

以下是完整的C语言代码,用于从现有文件中提取其IP数据包中的证书,并写入cer文件:
```
#include <openssl/bio.h>
#include <openssl/ssl.h>
#include <openssl/err.h>
#include <openssl/pem.h>
#include <stdint.h>
BIO *certbio = NULL;
X509 *cert = NULL;
FILE *pcapfile = NULL;
FILE *cerfile = NULL;
// 定义TLS协议头结构体
typedef struct {
uint8_t type;
uint8_t major;
uint8_t minor;
uint16_t length;
} tls_header_t;
// 定义TLS握手协议头结构体
typedef struct {
uint8_t type;
uint8_t length[3];
} tls_handshake_header_t;
int main()
{
// 打开pcap文件,并读取IP数据包
pcapfile = fopen("test.pcap", "rb");
if (pcapfile != NULL) {
// 跳过pcap文件头
fseek(pcapfile, 24, SEEK_SET);
// 读取IP数据包
char packet[65535];
while (fread(packet, 1, 65535, pcapfile) > 0) {
// 判断是否是TLS协议
if (packet[23] == 0x17) {
// 解析TLS协议,提取证书信息
tls_handshake_header_t *handshake_header = (tls_handshake_header_t *)(&packet[54]);
uint32_t cert_len = (handshake_header->length[0] << 16) | (handshake_header->length[1] << 8) | handshake_header->length[2];
certbio = BIO_new(BIO_s_mem());
BIO_write(certbio, &packet[58], cert_len);
cert = PEM_read_bio_X509(certbio, NULL, 0, NULL);
// 将证书信息写入cer文件
cerfile = fopen("cert.cer", "wb");
if (cerfile != NULL) {
i2d_X509_fp(cerfile, cert);
fclose(cerfile);
}
// 释放资源
X509_free(cert);
BIO_free_all(certbio);
}
}
fclose(pcapfile); // 关闭文件指针
}
return 0;
}
```
其中,test.pcap是pcap文件的文件名,cert.cer是要写入的cer文件名。在编译之前,需要确保已经安装了OpenSSL库,可以使用以下命令进行安装:
```
sudo apt-get install libssl-dev
```
编译命令如下:
```
gcc -o extract_cert extract_cert.c -lcrypto -lssl
```
运行命令如下:
```
./extract_cert
```
运行后,程序会自动从pcap文件中提取证书信息,并写入cer文件。
阅读全文
相关推荐
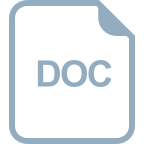
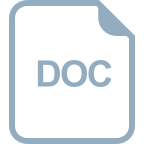




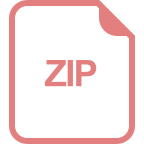
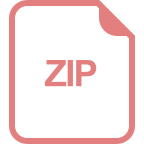
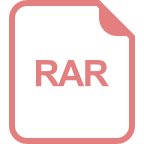








