从现有IP数据文件中提取所有的证书并写入cer文件 c语言
时间: 2024-03-05 19:51:26 浏览: 22
要实现该功能,可以按照以下步骤进行:
1. 打开IP数据文件并读取其中的数据。
2. 遍历数据,查找所有的证书信息。
3. 将证书信息写入一个cer文件中。
下面是一个简单的示例代码,可以帮助你完成该功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_CERT_LENGTH 4096
int main()
{
FILE* ip_file = fopen("ip_data.txt", "r");
FILE* cer_file = fopen("certs.cer", "wb");
char cert_buffer[MAX_CERT_LENGTH];
memset(cert_buffer, 0, MAX_CERT_LENGTH);
int cert_count = 0;
if (ip_file == NULL || cer_file == NULL) {
printf("Failed to open file\n");
return 1;
}
while (!feof(ip_file)) {
char line_buffer[1024];
fgets(line_buffer, 1024, ip_file);
// Check if this line contains a certificate
if (strstr(line_buffer, "CERTIFICATE") != NULL) {
// Copy the certificate data to the buffer
strcat(cert_buffer, line_buffer);
// Increase the certificate counter
cert_count++;
}
}
// Write the certificate data to the cer file
fwrite(cert_buffer, sizeof(char), strlen(cert_buffer), cer_file);
printf("Extracted %d certificates\n", cert_count);
fclose(ip_file);
fclose(cer_file);
return 0;
}
```
在该代码中,我们从ip_data.txt文件中读取数据,并遍历数据以查找所有的证书信息。一旦找到证书信息,我们就将其存储在cert_buffer数组中,并增加证书计数器cert_count。最后,我们将cert_buffer数组中的数据写入到一个新的cer文件中,并打印出提取的证书数量。
请注意,这只是一个简单的示例代码,实际情况可能会更加复杂。例如,证书可能会跨越多行,需要特殊处理。此外,该代码也没有对证书数据进行任何验证或解析,因此在实际应用中需要根据需要进行相应的处理。
相关推荐
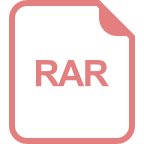














