Qt串口接收发送图片完整项目代码
时间: 2023-08-03 08:32:24 浏览: 108
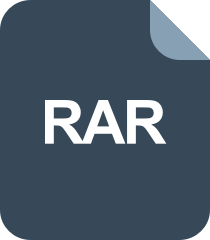
Qt串口传输图片

以下是一个完整的 Qt 项目代码,用于通过串口接收和发送图片。该项目包含一个 GUI 界面,可以选择串口号、波特率等参数,并且可以通过按钮进行图片的传输。你可以将以下代码保存为一个 .pro 文件,然后使用 Qt Creator 等工具进行编译和运行。
```
QT += core gui serialport
CONFIG += c++11
TARGET = SerialImageTransfer
TEMPLATE = app
SOURCES += main.cpp \
mainwindow.cpp
HEADERS += mainwindow.h
FORMS += mainwindow.ui
```
mainwindow.h 文件:
```cpp
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include <QSerialPort>
#include <QSerialPortInfo>
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
void on_selectButton_clicked();
void on_sendButton_clicked();
void on_receiveButton_clicked();
private:
Ui::MainWindow *ui;
QSerialPort *serial;
QByteArray imageData;
bool isReceiving;
void updatePortList();
};
#endif // MAINWINDOW_H
```
mainwindow.cpp 文件:
```cpp
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include <QFileDialog>
#include <QMessageBox>
#include <QDataStream>
#include <QImage>
#include <QDebug>
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
serial = new QSerialPort(this);
isReceiving = false;
updatePortList();
}
MainWindow::~MainWindow()
{
delete ui;
delete serial;
}
void MainWindow::updatePortList()
{
ui->portComboBox->clear();
QList<QSerialPortInfo> portList = QSerialPortInfo::availablePorts();
for (const QSerialPortInfo &portInfo : portList) {
ui->portComboBox->addItem(portInfo.portName());
}
}
void MainWindow::on_selectButton_clicked()
{
if (serial->isOpen()) {
serial->close();
ui->selectButton->setText(tr("Connect"));
} else {
QString portName = ui->portComboBox->currentText();
if (portName.isEmpty()) {
QMessageBox::warning(this, tr("Warning"), tr("Please select a serial port"));
return;
}
serial->setPortName(portName);
if (!serial->open(QIODevice::ReadWrite)) {
QMessageBox::warning(this, tr("Warning"), tr("Failed to open serial port"));
return;
}
serial->setBaudRate(ui->baudrateComboBox->currentText().toInt());
serial->setDataBits(QSerialPort::Data8);
serial->setParity(QSerialPort::NoParity);
serial->setStopBits(QSerialPort::OneStop);
serial->setFlowControl(QSerialPort::NoFlowControl);
ui->selectButton->setText(tr("Disconnect"));
}
}
void MainWindow::on_sendButton_clicked()
{
QString fileName = QFileDialog::getOpenFileName(this, tr("Open Image"), ".", tr("Image Files (*.png *.jpg *.bmp)"));
if (fileName.isEmpty()) {
return;
}
QImage image(fileName);
if (image.isNull()) {
QMessageBox::warning(this, tr("Warning"), tr("Failed to load image"));
return;
}
QByteArray imageData;
QDataStream stream(&imageData, QIODevice::ReadWrite);
stream << image;
if (serial->write(imageData) == -1) {
QMessageBox::warning(this, tr("Warning"), tr("Failed to send image"));
return;
}
QMessageBox::information(this, tr("Information"), tr("Image sent successfully"));
}
void MainWindow::on_receiveButton_clicked()
{
if (!isReceiving) {
imageData.clear();
isReceiving = true;
ui->receiveButton->setText(tr("Stop"));
} else {
isReceiving = false;
ui->receiveButton->setText(tr("Receive"));
QImage image;
if (image.loadFromData(imageData)) {
ui->imageLabel->setPixmap(QPixmap::fromImage(image));
} else {
QMessageBox::warning(this, tr("Warning"), tr("Failed to load image"));
}
}
}
void MainWindow::serialReceived()
{
while (serial->bytesAvailable() > 0) {
QByteArray data = serial->readAll();
imageData.append(data);
}
}
```
mainwindow.ui 文件:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<ui version="4.0">
<class>MainWindow</class>
<widget class="QMainWindow" name="MainWindow">
<property name="geometry">
<rect>
<x>0</x>
<y>0</y>
<width>400</width>
<height>300</height>
</rect>
</property>
<property name="windowTitle">
<string>Serial Image Transfer</string>
</property>
<widget class="QWidget" name="centralwidget">
<widget class="QLabel" name="imageLabel">
<property name="geometry">
<rect>
<x>10</x>
<y>10</y>
<width>380</width>
<height>220</height>
</rect>
</property>
<property name="frameShape">
<enum>QFrame::StyledPanel</enum>
</property>
<property name="text">
<string/>
</property>
<property name="scaledContents">
<bool>true</bool>
</property>
</widget>
<widget class="QGroupBox" name="portGroupBox">
<property name="geometry">
<rect>
<x>10</x>
<y>240</y>
<width>150</width>
<height>50</height>
</rect>
</property>
<property name="title">
<string>Serial Port Settings</string>
</property>
<widget class="QComboBox" name="portComboBox">
<property name="geometry">
<rect>
<x>10</x>
<y>20</y>
<width>80</width>
<height>22</height>
</rect>
</property>
</widget>
<widget class="QComboBox" name="baudrateComboBox">
<property name="geometry">
<rect>
<x>100</x>
<y>20</y>
<width>40</width>
<height>22</height>
</rect>
</property>
<item>
<property name="text">
<string>9600</string>
</property>
</item>
<item>
<property name="text">
<string>19200</string>
</property>
</item>
<item>
<property name="text">
<string>38400</string>
</property>
</item>
<item>
<property name="text">
<string>57600</string>
</property>
</item>
<item>
<property name="text">
<string>115200</string>
</property>
</item>
</widget>
<widget class="QPushButton" name="selectButton">
<property name="geometry">
<rect>
<x>10</x>
<y>40</y>
<width>80</width>
<height>22</height>
</rect>
</property>
<property name="text">
<string>Connect</string>
</property>
</widget>
</widget>
<widget class="QGroupBox" name="imageGroupBox">
<property name="geometry">
<rect>
<x>170</x>
<y>240</y>
<width>150</width>
<height>50</height>
</rect>
</property>
<property name="title">
<string>Image</string>
</property>
<widget class="QPushButton" name="sendButton">
<property name="geometry">
<rect>
<x>10</x>
<y>20</y>
<width>60</width>
<height>22</height>
</rect>
</property>
<property name="text">
<string>Send</string>
</property>
</widget>
<widget class="QPushButton" name="receiveButton">
<property name="geometry">
<rect>
<x>80</x>
<y>20</y>
<width>60</width>
<height>22</height>
</rect>
</property>
<property name="text">
<string>Receive</string>
</property>
</widget>
</widget>
</widget>
<widget class="QMenuBar" name="menubar">
<property name="geometry">
<rect>
<x>0</x>
<y>0</y>
<width>400</width>
<height>21</height>
</rect>
</property>
</widget>
<widget class="QStatusBar" name="statusbar"/>
</widget>
<resources/>
<connections/>
</ui>
```
请注意,以上代码只是一个简单的示例,实际应用中需要根据具体需求进行修改和完善。同时,由于该项目涉及到 GUI 界面和串口通信等多个方面,因此需要一定的 Qt 编程经验才能理解和修改。
阅读全文
相关推荐
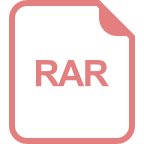
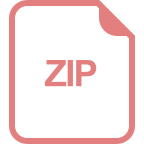
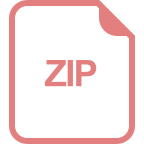
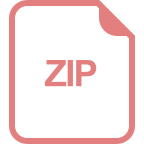
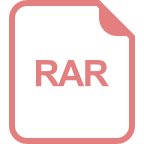
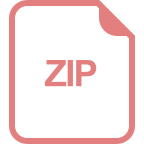
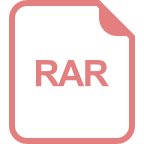
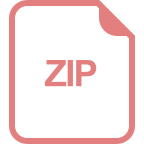
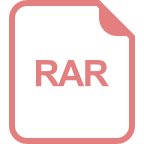
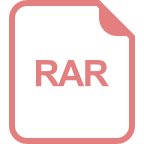
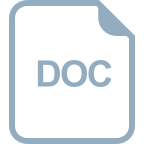
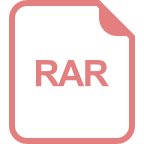
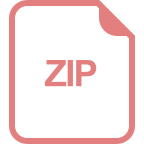
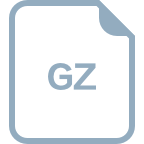
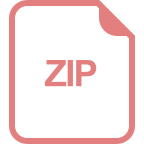
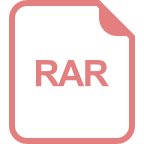