用C语言创建一个单链表
时间: 2023-04-03 19:03:53 浏览: 52
你好,以下是用 C 语言创建一个单链表的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data; // 数据域
struct Node *next; // 指针域
} Node;
// 创建链表函数
Node* createList(int n) {
Node *head = NULL, *tail = NULL; // 头指针和尾指针
int i, data;
for (i = 0; i < n; i++) {
printf("请输入第 %d 个节点的值:", i + 1);
scanf("%d", &data);
Node *newNode = (Node*)malloc(sizeof(Node)); // 创建新节点
newNode->data = data; // 赋值
newNode->next = NULL;
if (head == NULL) { // 如果是第一个节点
head = newNode;
tail = newNode;
} else {
tail->next = newNode; // 将新节点链接到链表尾部
tail = newNode; // 更新尾指针
}
}
return head; // 返回头指针
}
// 遍历链表函数
void traverseList(Node *head) {
Node *p = head;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
int n;
printf("请输入链表节点个数:");
scanf("%d", &n);
Node *head = createList(n); // 创建链表
printf("链表节点值为:");
traverseList(head); // 遍历链表
return 0;
}
```
希望能对你有所帮助。
相关推荐
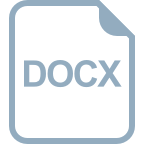
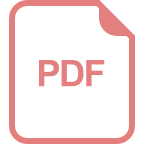














