用c语言创建一个单链表
时间: 2024-03-13 18:22:19 浏览: 21
以下是一个简单的单链表的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点
struct Node {
int data;
struct Node* next;
};
// 插入节点到链表头部
void insertAtBeginning(struct Node** head, int data) {
struct Node* new_node = (struct Node*) malloc(sizeof(struct Node));
new_node->data = data;
new_node->next = *head;
*head = new_node;
}
// 遍历链表并打印节点数据
void printList(struct Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
}
int main() {
struct Node* head = NULL;
// 插入节点
insertAtBeginning(&head, 3);
insertAtBeginning(&head, 2);
insertAtBeginning(&head, 1);
// 遍历链表并打印节点数据
printList(head);
return 0;
}
```
这个程序创建了一个简单的单链表,并在链表头部插入了三个节点。使用 `insertAtBeginning` 函数插入节点,该函数将创建一个新节点并将其插入到链表的头部。使用 `printList` 函数遍历链表并打印节点数据。
相关推荐
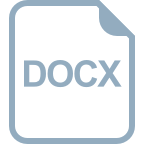
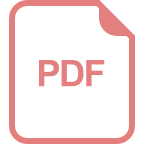














