停车场管理系统数据结构的定义
时间: 2023-07-11 22:56:39 浏览: 114
停车场管理系统通常包含以下数据结构的定义:
1. 停车场:表示一个停车场,包含停车位、收费标准等信息。
2. 停车位:表示停车场中的一个停车位,包含车位编号、车位状态(是否空闲)等信息。
3. 车辆:表示一辆车,包含车牌号码、车型、入场时间等信息。
4. 停车记录:表示一条停车记录,包含车辆信息、入场时间、出场时间、停车费用等信息。
5. 收费标准:表示停车场的收费标准,包含收费方式、收费规则等信息。
6. 收费记录:表示一条收费记录,包含车辆信息、收费时间、收费金额等信息。
以上是停车场管理系统中常见的数据结构定义,不同的系统可能会有所不同。
相关问题
停车场管理系统数据结构C语言
停车场管理系统数据结构可以用C语言实现,以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_CARS 50
struct Car {
char license_plate[10];
int entry_time;
int exit_time;
};
struct ParkingLot {
struct Car cars[MAX_CARS];
int num_cars;
};
void add_car(struct ParkingLot *parking_lot, char *license_plate, int entry_time) {
if (parking_lot->num_cars >= MAX_CARS) {
printf("Parking lot is full.\n");
return;
}
struct Car car;
strcpy(car.license_plate, license_plate);
car.entry_time = entry_time;
car.exit_time = -1;
parking_lot->cars[parking_lot->num_cars] = car;
parking_lot->num_cars++;
printf("Car with license plate %s added to the parking lot.\n", license_plate);
}
void remove_car(struct ParkingLot *parking_lot, char *license_plate, int exit_time) {
int i;
for (i = 0; i < parking_lot->num_cars; i++) {
if (strcmp(parking_lot->cars[i].license_plate, license_plate) == 0) {
if (parking_lot->cars[i].exit_time != -1) {
printf("Car with license plate %s has already left the parking lot.\n", license_plate);
} else {
parking_lot->cars[i].exit_time = exit_time;
printf("Car with license plate %s has left the parking lot.\n", license_plate);
}
return;
}
}
printf("Car with license plate %s not found in the parking lot.\n", license_plate);
}
void print_parking_lot(struct ParkingLot *parking_lot) {
int i;
for (i = 0; i < parking_lot->num_cars; i++) {
printf("Car with license plate %s entered at %d", parking_lot->cars[i].license_plate, parking_lot->cars[i].entry_time);
if (parking_lot->cars[i].exit_time != -1) {
printf(" and exited at %d", parking_lot->cars[i].exit_time);
}
printf("\n");
}
}
int main() {
struct ParkingLot parking_lot;
parking_lot.num_cars = 0;
add_car(&parking_lot, "ABC123", 1000);
add_car(&parking_lot, "DEF456", 1030);
add_car(&parking_lot, "GHI789", 1100);
remove_car(&parking_lot, "DEF456", 1200);
print_parking_lot(&parking_lot);
return 0;
}
```
这个示例中,我们使用了两个结构体:`Car` 和 `ParkingLot`。`Car` 结构体包含车牌号、进入时间和离开时间。`ParkingLot` 结构体包含一个 `Car` 数组和当前停车场中汽车的数量。
我们定义了三个函数:`add_car`、`remove_car` 和 `print_parking_lot`。`add_car` 函数用于添加车辆到停车场,`remove_car` 函数用于移除停车场中的车辆,`print_parking_lot` 函数用于打印当前停车场中的所有车辆信息。
在 `main` 函数中,我们创建了一个 `ParkingLot` 结构体,并使用 `add_car` 函数添加了三辆车。然后,我们使用 `remove_car` 函数将一辆车移除,并使用 `print_parking_lot` 函数打印当前停车场中所有车辆的信息。
这个示例只是一个简单的停车场管理系统的数据结构,实际应用中可能需要更复杂的功能和更复杂的数据结构。
停车场管理系统数据结构C++
停车场管理系统可以使用栈或队列来实现。下面以使用队列为例:
1. 定义车辆结构体:
```cpp
struct Car {
string licensePlate; // 车牌号
int arrivalTime; // 到达时间
};
```
2. 定义停车场类,其中包含入场、出场、查询等操作:
```cpp
class ParkingLot {
private:
queue<Car> cars; // 停车场队列
int capacity; // 停车场容量
int feeRate; // 收费标准
public:
ParkingLot(int cap, int rate) : capacity(cap), feeRate(rate) {}
bool park(Car car); // 入场
int leave(string licensePlate); // 出场
int query(string licensePlate); // 查询
};
```
3. 实现入场操作:
```cpp
bool ParkingLot::park(Car car) {
if (cars.size() == capacity) return false; // 停车场已满
cars.push(car);
return true;
}
```
4. 实现出场操作:
```cpp
int ParkingLot::leave(string licensePlate) {
int fee = 0;
queue<Car> temp; // 临时队列
while (!cars.empty()) {
Car car = cars.front();
cars.pop();
if (car.licensePlate == licensePlate) { // 找到车辆
fee = (time(NULL) - car.arrivalTime) * feeRate; // 计算费用
break;
}
temp.push(car);
}
while (!temp.empty()) { // 将临时队列中的车辆重新加入停车场队列
cars.push(temp.front());
temp.pop();
}
return fee;
}
```
5. 实现查询操作:
```cpp
int ParkingLot::query(string licensePlate) {
int time = -1;
queue<Car> temp; // 临时队列
while (!cars.empty()) {
Car car = cars.front();
cars.pop();
if (car.licensePlate == licensePlate) { // 找到车辆
time = time(NULL) - car.arrivalTime; // 计算停车时间
break;
}
temp.push(car);
}
while (!temp.empty()) { // 将临时队列中的车辆重新加入停车场队列
cars.push(temp.front());
temp.pop();
}
return time;
}
```
以上就是一个简单的停车场管理系统数据结构的实现。
阅读全文
相关推荐
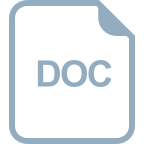
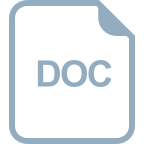
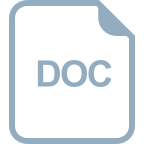
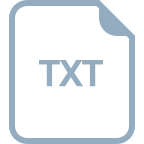
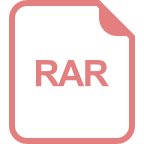
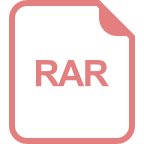
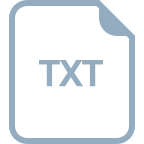
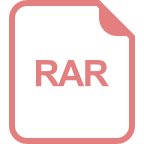
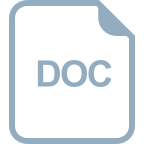
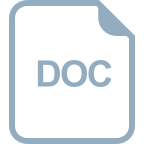
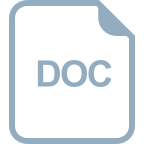
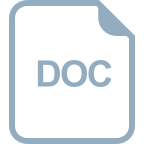
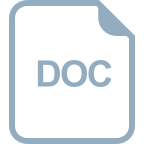