通过MapReduce将三张表格信息根据关联字段进行合并处理代码
时间: 2023-07-31 19:06:38 浏览: 51
假设我们有三张表格,分别为A表、B表和C表,它们的关联字段分别为a_id、b_id和c_id。我们需要将它们的相关信息合并到一个新的表格中,可以通过MapReduce来实现。
下面是一个简单的MapReduce代码示例:
```java
// Mapper类
public class JoinMapper extends Mapper<LongWritable, Text, Text, Text> {
private Text mapOutputKey = new Text();
private Text mapOutputValue = new Text();
@Override
protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] fields = value.toString().split(",");
String table = fields[0]; // 表格名称
String id = fields[1]; // 关联字段值
String data = fields[2]; // 数据内容
// 根据表格名称和关联字段值生成Map输出的Key和Value
mapOutputKey.set(id);
mapOutputValue.set(table + "," + data);
context.write(mapOutputKey, mapOutputValue);
}
}
// Reducer类
public class JoinReducer extends Reducer<Text, Text, Text, Text> {
private Text outputValue = new Text();
@Override
protected void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
StringBuilder sb = new StringBuilder();
String prefix = "";
for (Text value : values) {
String[] fields = value.toString().split(",");
String table = fields[0]; // 表格名称
String data = fields[1]; // 数据内容
sb.append(prefix).append(table).append(":").append(data);
prefix = ",";
}
outputValue.set(sb.toString());
context.write(key, outputValue);
}
}
// 主函数
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "Join Tables");
job.setJarByClass(JoinTables.class);
job.setMapperClass(JoinMapper.class);
job.setReducerClass(JoinReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
```
上述代码中,Mapper类用于将每个表格的数据转换为Map输出的Key-Value键值对,其中Key为关联字段值,Value为“表格名称,数据内容”的组合。Reducer类用于将每个关联字段的所有数据合并为一条记录,其中Value为“表格名称:数据内容,表格名称:数据内容,...”的组合。主函数中设置了输入和输出路径,并且将Mapper和Reducer类设置为任务的处理类。
需要注意的是,以上代码示例仅供参考,具体实现需要根据具体的数据和环境进行调整和优化。
相关推荐
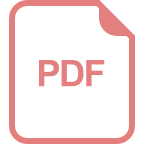
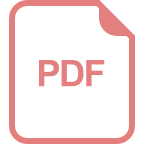














