python 向json追加字典
时间: 2023-07-06 20:24:17 浏览: 85
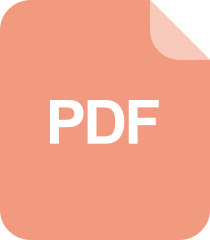
python的json包位置及用法总结
可以使用 `json` 和 `os` 模块中的函数来完成向 `json` 文件中追加字典的操作。具体步骤如下:
1. 读取 `json` 文件中已有的数据,将其转换成字典或列表类型。
2. 向字典或列表类型中添加新的数据。
3. 将更新后的数据写回到 `json` 文件中。
以下是一个示例代码:
```python
import json
import os
# 读取 json 文件中已有的数据
if os.path.exists('data.json'):
with open('data.json', 'r') as f:
data = json.load(f)
else:
data = []
# 向字典或列表类型中添加新的数据
new_data = {'name': 'Alice', 'age': 25}
data.append(new_data)
# 将更新后的数据写回到 json 文件中
with open('data.json', 'w') as f:
json.dump(data, f)
```
运行以上代码后,如果 `data.json` 文件存在,则会在原有数据的基础上添加新的数据;如果 `data.json` 文件不存在,则会创建一个新的文件,并将新的数据写入其中。
阅读全文
相关推荐
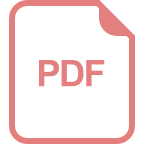
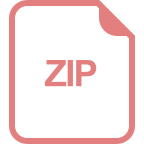















