C#大文件流式压缩加解密
时间: 2023-08-03 14:21:46 浏览: 59
对于大文件的流式压缩和加解密,我可以给你一些思路和建议:
1. 压缩:可以使用压缩算法如GZIP、ZIP等对大文件进行流式压缩。在C#中,可以使用System.IO.Compression命名空间提供的GZipStream和ZipArchive类来实现。
示例代码:
```csharp
using (var sourceStream = File.OpenRead(sourceFilePath))
{
using (var targetStream = File.Create(targetFilePath))
{
using (var gzipStream = new GZipStream(targetStream, CompressionMode.Compress))
{
sourceStream.CopyTo(gzipStream);
}
}
}
```
2. 解压:对于经过压缩的大文件,同样可以使用GZipStream或ZipArchive类进行流式解压缩。
示例代码:
```csharp
using (var sourceStream = File.OpenRead(sourceFilePath))
{
using (var targetStream = File.Create(targetFilePath))
{
using (var gzipStream = new GZipStream(sourceStream, CompressionMode.Decompress))
{
gzipStream.CopyTo(targetStream);
}
}
}
```
3. 加密:对于大文件的加密,可以使用加密算法如AES、DES等对大文件进行分块加密,以保证加密效率和安全性。在C#中,可以使用System.Security.Cryptography命名空间提供的加密类来实现。
示例代码:
```csharp
using (var sourceStream = File.OpenRead(sourceFilePath))
{
using (var targetStream = File.Create(targetFilePath))
{
using (var aes = Aes.Create())
{
// 设置加密密钥和向量
aes.Key = key;
aes.IV = iv;
// 创建加密流
using (var encryptor = aes.CreateEncryptor())
{
using (var cryptoStream = new CryptoStream(targetStream, encryptor, CryptoStreamMode.Write))
{
sourceStream.CopyTo(cryptoStream);
}
}
}
}
}
```
4. 解密:对于经过加密的大文件,同样可以使用加密类进行分块解密。
示例代码:
```csharp
using (var sourceStream = File.OpenRead(sourceFilePath))
{
using (var targetStream = File.Create(targetFilePath))
{
using (var aes = Aes.Create())
{
// 设置解密密钥和向量
aes.Key = key;
aes.IV = iv;
// 创建解密流
using (var decryptor = aes.CreateDecryptor())
{
using (var cryptoStream = new CryptoStream(sourceStream, decryptor, CryptoStreamMode.Read))
{
cryptoStream.CopyTo(targetStream);
}
}
}
}
}
```
需要注意的是,在进行大文件的流式处理时,应该尽量避免将整个文件读取到内存中,而是采用分块读取的方式,以减少内存占用和提高处理效率。同时,在进行加解密操作时,应该谨慎处理加密密钥和向量,以保证数据的安全性。
相关推荐
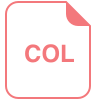













