python mean
时间: 2023-09-09 08:04:30 浏览: 73
### 回答1:
In Python, "mean" refers to the average value of a list of numbers. You can calculate the mean using the following code:
```python
def mean(numbers):
return sum(numbers) / len(numbers)
```
Here, "numbers" is a list of numerical values. The function first calculates the sum of all the numbers in the list using the `sum()` function, and then divides that sum by the length of the list to get the mean value.
You can call this function with your list of numbers like this:
```python
my_list = [1, 2, 3, 4, 5]
print(mean(my_list)) # Output: 3.0
```
This will output the mean of the numbers in the list, which is 3 in this case.
### 回答2:
Python中的mean是指计算一组数字的平均值。平均值是将一组数字的总和除以数字的个数得到的结果。在Python中,可以使用numpy库的mean函数来计算平均值,也可以手动编写代码来实现。
例如,我们有一组数字[1, 2, 3, 4, 5],我们想要计算它们的平均值。我们可以使用numpy库的mean函数来实现:
```python
import numpy as np
numbers = [1, 2, 3, 4, 5]
mean = np.mean(numbers)
print(mean) # 输出结果为3.0
```
在这个例子中,我们将数字列表传递给np.mean()函数,并将返回的平均值存储在mean变量中。最后,我们使用print()函数来输出结果。
如果我们想手动编写代码来计算平均值,我们可以使用for循环来遍历数字列表,并将每个数字累加起来。然后我们将得到的总和除以数字的个数即可得到平均值。以下是手动实现的代码:
```python
numbers = [1, 2, 3, 4, 5]
total = 0
for num in numbers:
total += num
mean = total / len(numbers)
print(mean) # 输出结果为3.0
```
在这个例子中,我们使用total变量来存储数字的累加和。然后我们使用len()函数获取数字的个数,并将total除以个数得到平均值。最后,我们使用print()函数来输出结果。
无论是使用numpy库的mean函数还是手动编写代码来计算平均值,都可以得到相同的结果。
### 回答3:
Python中的mean(平均值)是一种统计计算方法,用于计算给定数据集中数值的平均数。在Python中,我们可以使用numpy或statistics等库中的函数来计算平均值。
以numpy库为例,可以使用numpy.mean()函数来计算平均值。这个函数可以接受一个数组作为参数,并返回数组中所有元素的平均值。具体操作如下:
1. 首先,导入numpy库:import numpy as np
2. 创建一个数组:arr = np.array([1, 2, 3, 4, 5])
3. 使用mean()函数计算平均值:average = np.mean(arr)
4. 打印结果:print(average)
上述代码将输出3.0,表示给定数组的平均值为3.0。
值得注意的是,mean()函数还可以接受其他参数,例如axis参数用于指定计算平均值的轴。在多维数组中,可以通过指定不同的轴来计算沿特定方向的平均值。
除了numpy库之外,python还提供了statistics库中的mean()函数用来计算平均值。使用方法类似,首先导入statistics库,然后创建数组,并使用mean()函数计算平均值。
综上所述,Python中的mean函数是用来计算给定数据集中数值的平均数的函数,可以使用numpy库或statistics库中的mean()函数来实现。
相关推荐















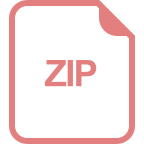
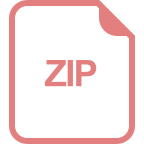