c语言用栈实现高精度整数求和
时间: 2023-07-13 18:07:05 浏览: 91
下面是基于栈实现高精度整数求和的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_N 1000
// 定义栈结构体
typedef struct {
int top;
int data[MAX_N];
} Stack;
// 初始化栈
void init(Stack *s) {
s->top = -1;
}
// 判断栈是否为空
int isEmpty(Stack *s) {
return s->top == -1;
}
// 入栈
void push(Stack *s, int x) {
s->data[++s->top] = x;
}
// 出栈
int pop(Stack *s) {
return s->data[s->top--];
}
// 高精度整数相加
void add(Stack *s1, Stack *s2, Stack *ans) {
int carry = 0; // 进位
while (!isEmpty(s1) || !isEmpty(s2)) {
int x = isEmpty(s1) ? 0 : pop(s1);
int y = isEmpty(s2) ? 0 : pop(s2);
int sum = x + y + carry;
push(ans, sum % 10);
carry = sum / 10;
}
if (carry > 0) {
push(ans, carry);
}
}
int main() {
char str1[MAX_N], str2[MAX_N];
Stack s1, s2, ans;
// 读入两个字符串
printf("请输入第一个整数:");
scanf("%s", str1);
printf("请输入第二个整数:");
scanf("%s", str2);
// 初始化栈
init(&s1);
init(&s2);
init(&ans);
// 字符串转换为栈
for (int i = 0; i < strlen(str1); i++) {
push(&s1, str1[i] - '0');
}
for (int i = 0; i < strlen(str2); i++) {
push(&s2, str2[i] - '0');
}
// 高精度整数相加
add(&s1, &s2, &ans);
// 输出结果
printf("相加的结果为:");
while (!isEmpty(&ans)) {
printf("%d", pop(&ans));
}
printf("\n");
return 0;
}
```
该程序中,我们定义了一个栈结构体,使用栈来存储每一位数字。输入的两个字符串通过遍历存储到两个栈中,然后对两个栈进行相加,结果存储在另一个栈中。最后,遍历结果栈,输出相加的结果。
阅读全文
相关推荐
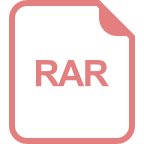
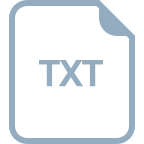

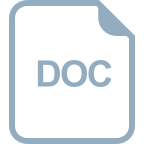
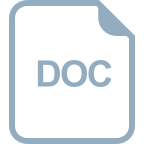


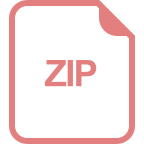
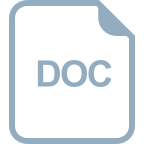
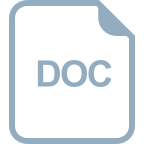
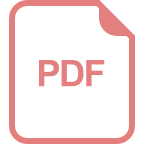
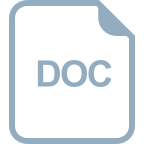
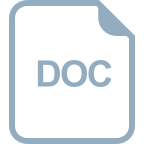
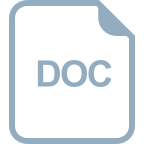
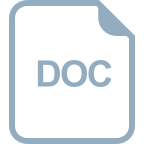
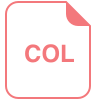


